附加动量的BP算法、学习率自适应算法和LM算法,其代表性的训练函数分别为
时间: 2023-11-10 11:06:44 浏览: 52
附加动量的BP算法的代表性训练函数为:
```python
def train_with_momentum(x_train, y_train, learning_rate, momentum, num_epochs):
# 初始化权重和偏置
w = np.random.randn(x_train.shape[1], 1)
b = np.random.randn()
# 初始化动量
v_w = np.zeros_like(w)
v_b = 0
# 训练
for epoch in range(num_epochs):
# 前向传播
z = np.dot(x_train, w) + b
a = sigmoid(z)
# 计算损失和梯度
loss = binary_cross_entropy(y_train, a)
d_loss = a - y_train
d_z = d_loss * sigmoid_derivative(a)
# 计算动量
v_w = momentum * v_w - learning_rate * np.dot(x_train.T, d_z)
v_b = momentum * v_b - learning_rate * np.sum(d_z)
# 更新权重和偏置
w += v_w
b += v_b
return w, b
```
学习率自适应算法的代表性训练函数为:
```python
def train_with_adaptive_learning_rate(x_train, y_train, num_epochs):
# 初始化权重和偏置
w = np.random.randn(x_train.shape[1], 1)
b = np.random.randn()
# 初始化学习率
learning_rate = 0.1
# 训练
for epoch in range(num_epochs):
# 前向传播
z = np.dot(x_train, w) + b
a = sigmoid(z)
# 计算损失和梯度
loss = binary_cross_entropy(y_train, a)
d_loss = a - y_train
d_z = d_loss * sigmoid_derivative(a)
# 更新权重和偏置
w -= learning_rate * np.dot(x_train.T, d_z)
b -= learning_rate * np.sum(d_z)
# 自适应学习率
if epoch % 10 == 0:
if loss < 0.1:
learning_rate *= 0.5
elif loss > 0.5:
learning_rate *= 1.1
return w, b
```
LM算法的代表性训练函数为:
```python
def train_with_lm(x_train, y_train, num_epochs):
# 初始化权重和偏置
w = np.random.randn(x_train.shape[1], 1)
b = np.random.randn()
# 初始化阻尼因子
mu = 0.1
# 训练
for epoch in range(num_epochs):
# 前向传播
z = np.dot(x_train, w) + b
a = sigmoid(z)
# 计算损失和梯度
loss = binary_cross_entropy(y_train, a)
d_loss = a - y_train
d_z = d_loss * sigmoid_derivative(a)
# 计算Hessian矩阵和雅可比矩阵
H = np.dot(x_train.T, x_train) + mu * np.eye(x_train.shape[1])
J = np.dot(x_train.T, d_z)
# 求解线性方程组
dw, db = np.linalg.solve(H, -J)
# 更新权重和偏置
w += dw.reshape(w.shape)
b += db
# 自适应阻尼因子
if loss < 0.1:
mu *= 0.5
elif loss > 0.5:
mu *= 2
return w, b
```
相关推荐
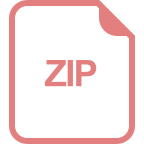
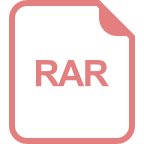












