pyqt主界面中显示子界面,等待子界面的enter按下时,子界面再close
时间: 2023-08-01 09:09:53 浏览: 154
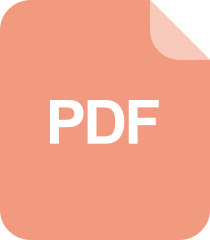
python使用pyqt写带界面工具的示例代码
你可以使用 PyQt 的 QDialog 类来实现子界面的显示和关闭。你可以在主界面中创建一个按钮或者其他交互元素,当用户点击时会触发一个事件,该事件会创建并显示子界面。
在子界面中,你可以监听按键事件,如果用户按下了 Enter 键,就可以关闭子界面并将结果返回给主界面。下面是一个简单的示例代码:
```
from PyQt5.QtWidgets import QApplication, QMainWindow, QDialog, QLabel, QVBoxLayout, QPushButton
from PyQt5.QtCore import Qt
class ChildDialog(QDialog):
def __init__(self, parent=None):
super().__init__(parent)
self.setWindowTitle('子界面')
self.layout = QVBoxLayout()
self.label = QLabel('请输入一些内容:')
self.text_input = QLineEdit()
self.btn_ok = QPushButton('确认')
self.btn_ok.clicked.connect(self.on_btn_ok_clicked)
self.layout.addWidget(self.label)
self.layout.addWidget(self.text_input)
self.layout.addWidget(self.btn_ok)
self.setLayout(self.layout)
def on_btn_ok_clicked(self):
self.accept() # 关闭子界面并返回结果
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('主界面')
self.btn_show_child = QPushButton('显示子界面')
self.btn_show_child.clicked.connect(self.on_btn_show_child_clicked)
self.setCentralWidget(self.btn_show_child)
def on_btn_show_child_clicked(self):
child_dialog = ChildDialog(self)
if child_dialog.exec_() == QDialog.Accepted:
text = child_dialog.text_input.text()
print('输入的内容为:', text)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在上面的代码中,我们创建了一个主界面 `MainWindow` 和一个子界面 `ChildDialog`。当用户点击主界面上的按钮时,会触发 `on_btn_show_child_clicked` 方法,该方法会创建并显示子界面。当用户在子界面中点击确认按钮时,子界面会关闭并返回结果给主界面。
请注意,在子界面中我们使用了 `accept` 方法来关闭对话框并返回结果,而不是使用 `close` 方法。这是因为 `accept` 方法会设置对话框的返回值为 `QDialog.Accepted`,而这个返回值会在 `exec_` 方法中返回给父窗口,以便我们知道用户是否点击了确认按钮。
阅读全文
相关推荐
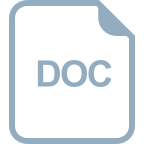
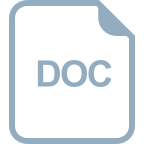
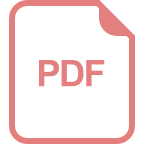
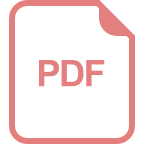
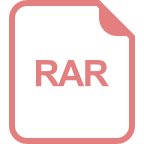
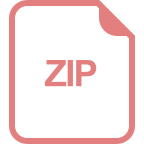
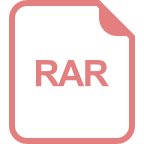
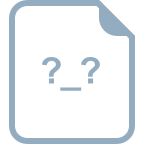
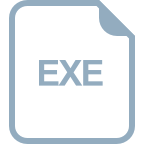
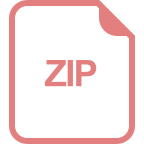
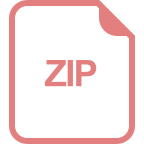
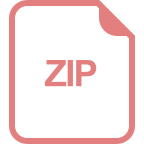
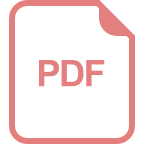
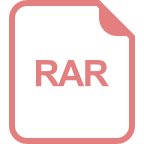
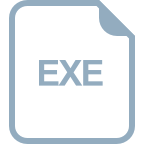