c语言实现des算法的代码
时间: 2024-10-23 15:05:05 浏览: 25
DES (Data Encryption Standard) 是一种已过时但仍然常见的对称加密算法。在 C 语言中实现 DES 算法通常需要大量的低级操作,因为这个算法涉及位操作、字节移位、异或等。以下是一个简化版的 DES 加密函数示例,它包含了基本的结构,但完整的 DES 实现会更复杂,并且通常不在生产环境中直接使用,而是通过安全库如 OpenSSL 来调用。
```c
#include <stdio.h>
#include <string.h>
#define KEY_SIZE 8 // DES key size in bytes
#define TABLES 48 // Number of S-boxes
// DES S-boxes and other constants
char des_sboxes[TABLES][256]; // S-box array
...
void expand_key(char key[], char expanded_key[KEY_SIZE * 4]) {
// Key expansion for DES
// (omitted for brevity)
}
void permute(const char input[], char output[]) {
// P-box permutation (also omitted for brevity)
}
void feistel(char block[], char round_key[]) {
int i;
for (i = 0; i < 16; i += 2) {
// Perform Feistel substitution
// (code involving XOR operations)
}
}
void des_encrypt(char plaintext[], char ciphertext[], char key[]) {
char temp_block[16];
expand_key(key, ...); // Expand the key
// Process the plaintext in 16-byte blocks
for (int i = 0; i <= 15; i++) {
memcpy(temp_block, plaintext + i * 16, 16);
feistel(temp_block, round_key + i * 16); // Apply Feistel function
// Combine with next block (XOR operation)
// (omitted for brevity)
memcpy(plaintext + i * 16, temp_block, 16);
}
}
阅读全文
相关推荐
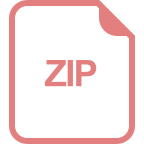
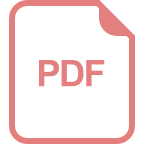






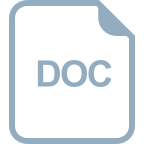
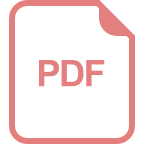
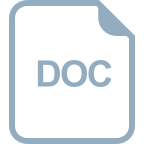
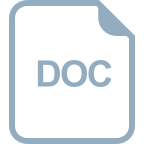

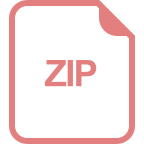
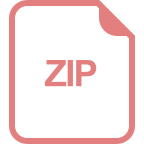