如何用C#创建一个学生信息系统
时间: 2024-05-08 11:21:50 浏览: 47
下面是一个简单的学生信息系统的示例代码,你可以在 Visual Studio 中使用 C# 来创建它:
```csharp
using System;
using System.Collections.Generic;
namespace StudentInfoSystem
{
class Program
{
static void Main(string[] args)
{
List<Student> students = new List<Student>();
while (true)
{
Console.WriteLine("请选择操作:");
Console.WriteLine("1. 添加学生");
Console.WriteLine("2. 显示所有学生");
Console.WriteLine("3. 退出");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
AddStudent(students);
break;
case "2":
ShowAllStudents(students);
break;
case "3":
return;
default:
Console.WriteLine("无效的输入,请重新输入。");
break;
}
}
}
static void AddStudent(List<Student> students)
{
Console.WriteLine("请输入学生信息:");
Console.Write("姓名:");
string name = Console.ReadLine();
Console.Write("年龄:");
int age = int.Parse(Console.ReadLine());
Console.Write("性别(男/女):");
string gender = Console.ReadLine();
Console.Write("学号:");
string id = Console.ReadLine();
students.Add(new Student(name, age, gender, id));
Console.WriteLine("学生信息添加成功!");
}
static void ShowAllStudents(List<Student> students)
{
if (students.Count == 0)
{
Console.WriteLine("当前没有学生信息。");
}
else
{
Console.WriteLine("所有学生信息如下:");
Console.WriteLine("------------------------");
foreach (Student student in students)
{
Console.WriteLine("姓名:" + student.Name);
Console.WriteLine("年龄:" + student.Age);
Console.WriteLine("性别:" + student.Gender);
Console.WriteLine("学号:" + student.Id);
Console.WriteLine("------------------------");
}
}
}
}
class Student
{
public string Name { get; set; }
public int Age { get; set; }
public string Gender { get; set; }
public string Id { get; set; }
public Student(string name, int age, string gender, string id)
{
Name = name;
Age = age;
Gender = gender;
Id = id;
}
}
}
```
这是一个简单的控制台应用程序,可以添加、显示学生信息以及退出程序。当你选择添加学生时,程序会提示你输入学生的姓名、年龄、性别和学号;当你选择显示所有学生时,程序会将所有学生的信息逐一输出到控制台上。
阅读全文
相关推荐
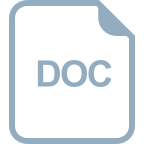
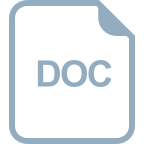
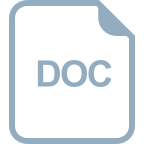
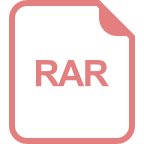
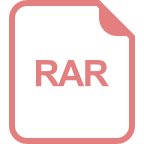
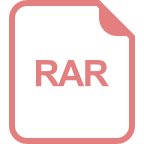
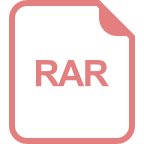
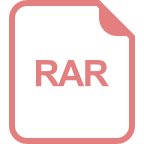
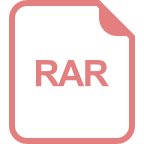
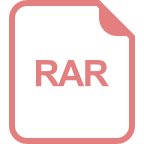
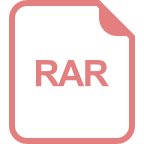
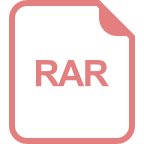
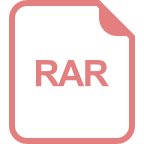
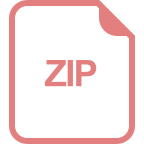
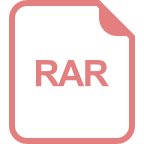
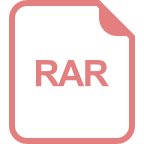
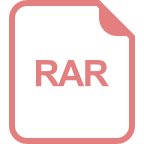
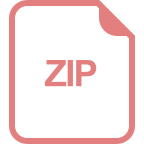
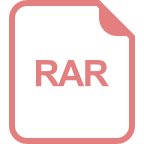