用python编写一道程序:输入一周有几天,一天有几个小时,一小时有几分钟,一分钟有多少秒。计算并输出一周有多少分钟,多少秒?
时间: 2024-03-18 08:39:54 浏览: 126
可以用以下的 Python 代码实现:
```python
days = int(input("一周有几天?"))
hours = int(input("一天有几个小时?"))
minutes = int(input("一小时有几分钟?"))
seconds = int(input("一分钟有多少秒?"))
total_hours = days * hours
total_minutes = total_hours * minutes
total_seconds = total_minutes * seconds
print("一周有", total_minutes, "分钟")
print("一周有", total_seconds, "秒")
```
运行程序后,会提示用户输入一周有几天、一天有几个小时、一小时有几分钟、一分钟有多少秒,然后计算出一周有多少分钟、多少秒,并输出结果。
相关问题
编写一段python代码,内容是输入一个总秒数,计算几小时零几分钟零几秒钟。
当然,这是一个简单的数学转换和时间单位转换的问题,你可以用Python编写如下的代码来实现:
```python
def convert_seconds_to_hours_minutes_seconds(total_seconds):
hours = total_seconds // 3600
remaining_seconds = total_seconds % 3600
minutes = remaining_seconds // 60
seconds = remaining_seconds % 60
return hours, minutes, seconds
total_seconds = int(input("请输入总秒数:"))
hours, minutes, seconds = convert_seconds_to_hours_minutes_seconds(total_seconds)
print(f"总共有 {hours} 小时, {minutes} 分钟, {seconds} 秒.")
# 相关问题--
1. 这段代码的时间单位转换是如何实现的?
2. 如果输入的秒数超过一天(86400秒),结果会怎么样?
3. 如何优化此函数使其更简洁易读?
python编写一个日历系统,设计一个时间类Time,包含一天中小时,分钟,秒的属性及其相关运算,设计一个日期类Date,数据成员应该包含但不限定为年,月,日,星期几等属性,设计菜单系统,根据用户输入显示题目描述中的显示功能
好的,我来为您解答。首先,我们需要定义一个时间类Time,代码如下:
```python
class Time:
def __init__(self, hour=0, minute=0, second=0):
self.hour = hour
self.minute = minute
self.second = second
def __str__(self):
return "{:02d}:{:02d}:{:02d}".format(self.hour, self.minute, self.second)
def __add__(self, other):
seconds = self.second + other.second
minutes = self.minute + other.minute
hours = self.hour + other.hour
if seconds >= 60:
seconds -= 60
minutes += 1
if minutes >= 60:
minutes -= 60
hours += 1
if hours >= 24:
hours -= 24
return Time(hours, minutes, seconds)
```
这里我们定义了一个时间类Time,包含小时、分钟、秒的属性和相关运算。其中,`__str__`方法用于将时间对象转换成字符串,`__add__`方法用于时间的加法运算。
接下来,我们需要定义一个日期类Date:
```python
class Date:
def __init__(self, year=1900, month=1, day=1):
self.year = year
self.month = month
self.day = day
def __str__(self):
return "{:04d}-{:02d}-{:02d}".format(self.year, self.month, self.day)
def day_of_week(self):
days = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"]
a = (14 - self.month) // 12
y = self.year - a
m = self.month + 12 * a - 2
d = (self.day + y + y // 4 - y // 100 + y // 400 + (31 * m) // 12) % 7
return days[d]
```
这里我们定义了一个日期类Date,包含年、月、日、星期几等属性。其中,`__str__`方法用于将日期对象转换成字符串,`day_of_week`方法用于计算日期对应的星期几。
最后,我们需要设计一个菜单系统,根据用户输入显示题目描述中的显示功能,代码如下:
```python
def main():
date = Date()
time = Time()
while True:
print("=" * 30)
print("1. Set Date")
print("2. Set Time")
print("3. Add Time")
print("4. Display Date")
print("5. Display Time")
print("6. Display Day of Week")
print("0. Exit")
print("=" * 30)
choice = input("Enter your choice: ")
if choice == "1":
year = int(input("Enter year: "))
month = int(input("Enter month: "))
day = int(input("Enter day: "))
date = Date(year, month, day)
elif choice == "2":
hour = int(input("Enter hour: "))
minute = int(input("Enter minute: "))
second = int(input("Enter second: "))
time = Time(hour, minute, second)
elif choice == "3":
hour = int(input("Enter hour to add: "))
minute = int(input("Enter minute to add: "))
second = int(input("Enter second to add: "))
add_time = Time(hour, minute, second)
time += add_time
elif choice == "4":
print("Date is", date)
elif choice == "5":
print("Time is", time)
elif choice == "6":
print("Day of Week is", date.day_of_week())
elif choice == "0":
break
else:
print("Invalid choice!")
```
在主函数中,我们使用了一个while循环,不断显示菜单并根据用户输入执行相应的操作,直到用户选择退出。
现在,我们已经完成了一个简单的日历系统的设计。您可以根据需要进行修改和完善。
阅读全文
相关推荐
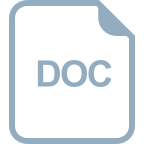
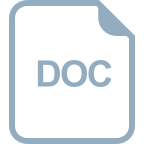
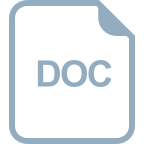
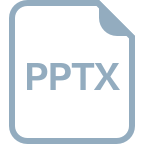
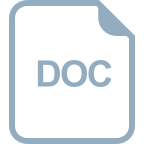
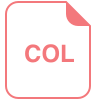
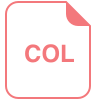
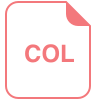
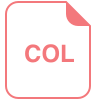
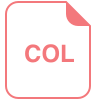
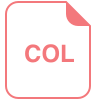
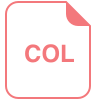
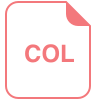
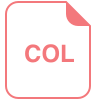
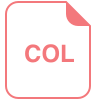
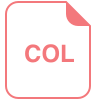