Java 给word的表格里面插入多张图片
时间: 2023-12-03 20:04:28 浏览: 66
要在 Word 表格中插入多张图片,可以使用 Java 的 Apache POI 库来实现。
以下是一个示例代码,它演示了如何向 Word 表格中插入两张图片:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableCell;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
public class InsertImagesToWordTable {
public static void main(String[] args) throws Exception {
XWPFDocument document = new XWPFDocument();
XWPFTable table = document.createTable(2, 2); // 创建一个 2 行 2 列的表格
List<XWPFTableRow> rows = table.getRows();
for (int i = 0; i < rows.size(); i++) {
XWPFTableRow row = rows.get(i);
List<XWPFTableCell> cells = row.getTableCells();
for (int j = 0; j < cells.size(); j++) {
XWPFTableCell cell = cells.get(j);
XWPFParagraph paragraph = cell.getParagraphs().get(0);
XWPFRun run = paragraph.createRun();
String imagePath = "image" + (i * 2 + j + 1) + ".jpg";
run.addPicture(new FileInputStream(imagePath), XWPFDocument.PICTURE_TYPE_JPEG, imagePath, 100, 100); // 插入图片
}
}
FileOutputStream out = new FileOutputStream("table_with_images.docx");
document.write(out);
out.close();
document.close();
}
}
```
该示例代码创建一个 2 行 2 列的表格,并在每个单元格中插入一张图片。在实际使用时,可以根据需要修改表格的行数和列数,以及图片的路径和大小。
相关推荐
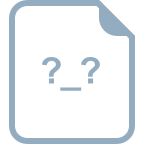














