Java实现对JSON字符串的压缩
时间: 2023-12-20 15:07:21 浏览: 222
可以使用Java中的GZIP压缩算法对JSON字符串进行压缩,具体实现步骤如下:
1. 将JSON字符串转换为字节数组。
2. 使用Java中的GZIPOutputStream对字节数组进行压缩,得到压缩后的字节数组。
3. 将压缩后的字节数组转换为Base64编码的字符串,以便于存储和传输。
下面是代码示例:
```java
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.Base64;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
public class JsonCompressor {
public static String compress(String json) throws IOException {
// 将JSON字符串转换为字节数组
byte[] jsonBytes = json.getBytes("UTF-8");
// 使用GZIP压缩算法对字节数组进行压缩
ByteArrayOutputStream byteOut = new ByteArrayOutputStream();
GZIPOutputStream gzipOut = new GZIPOutputStream(byteOut);
gzipOut.write(jsonBytes);
gzipOut.flush();
gzipOut.close();
// 将压缩后的字节数组转换为Base64编码的字符串
byte[] compressedBytes = byteOut.toByteArray();
String compressedJson = Base64.getEncoder().encodeToString(compressedBytes);
return compressedJson;
}
public static String decompress(String compressedJson) throws IOException {
// 将Base64编码的字符串转换为压缩后的字节数组
byte[] compressedBytes = Base64.getDecoder().decode(compressedJson);
// 使用GZIP压缩算法对字节数组进行解压缩
ByteArrayInputStream byteIn = new ByteArrayInputStream(compressedBytes);
GZIPInputStream gzipIn = new GZIPInputStream(byteIn);
ByteArrayOutputStream byteOut = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = gzipIn.read(buffer)) > 0) {
byteOut.write(buffer, 0, len);
}
gzipIn.close();
// 将解压缩后的字节数组转换为JSON字符串
byte[] jsonBytes = byteOut.toByteArray();
String json = new String(jsonBytes, "UTF-8");
return json;
}
}
```
使用示例:
```java
String json = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
String compressedJson = JsonCompressor.compress(json);
System.out.println(compressedJson); // "H4sIAAAAAAAAAGvMywEAEUjwWQAAAA=="
String decompressedJson = JsonCompressor.decompress(compressedJson);
System.out.println(decompressedJson); // "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}"
```
相关推荐
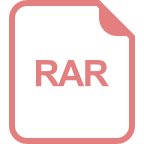
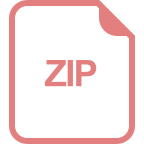














