ui->customPlot->yAxis2->setNumberFormat 这个接口怎么用
时间: 2024-10-19 09:12:08 浏览: 27
`ui->customPlot->yAxis2->setNumberFormat` 是一个Qt C++中的方法,用于设置QCustomPlot库(一种数据可视化工具包)中自定义绘图区域(custom plot)的第二个Y轴(yAxis2)的数字格式。在这个上下文中,`ui`通常是一个包含QCustomPlot实例的用户界面元素。
这个步骤通常是这样的:
1. 首先,你需要创建并初始化一个`QCustomPlot`对象,并将其添加到UI控件上。
```cpp
QCustomPlot *customPlot = new QCustomPlot(ui);
ui->customPlotLayout->addWidget(customPlot);
```
2. 然后,获取`yAxis2`的引用。`yAxis2`可能是通过布局或者其他方式设置的,如果没有明确指定,它可能默认存在。
```cpp
QCPAxis *yAxis2 = customPlot->axis(YLeft);
```
3. 最后,你可以调用`setNumberFormat`方法,传入一个格式字符串来定义显示的数值格式。例如,如果你想展示小数点后两位,可以这样做:
```cpp
QString format = "0.##";
yAxis2->setNumberFormat(format);
```
`format`可以是你需要的任何合法的Qt数字格式字符串,比如`#,###.###`、`%.2f`等。
相关问题
QCPAxis *yAxis = ui->customPlot->yAxis; double minY = std::numeric_limits<double>::max(); double maxY = std::numeric_limits<double>::min(); for (int i = 0; i < ui->customPlot->graphCount(); i++) { QCPGraph *graph = ui->customPlot->graph(i); if (graph->dataCount() == 0) { continue; } QCPGraphDataContainer::const_iterator it = graph->data().constBegin(); for (; it != graph->data().constEnd(); ++it) { double value = it->value; if (value < minY) { minY = value; } if (value > maxY) { maxY = value; } } } double range = qMax(qAbs(minY), qAbs(maxY)) * 2; double center = (minY + maxY) / 2; yAxis->setRange(center - range, center + range); 多条y轴,不是所有曲线共用y轴
如果多条曲线不是共用一个y轴,那么你需要对每个y轴分别进行自适应范围的计算和设置。
具体步骤如下:
1. 获取所有y轴的指针,可以通过QCustomPlot::axisRect()的成员函数axis()来获取。
```cpp
QList<QCPAxis*> yAxisList;
for (int i = 0; i < ui->customPlot->axisRectCount(); i++) {
QCPAxisRect *axisRect = ui->customPlot->axisRect(i);
QCPAxis *yAxis = axisRect->axis(QCPAxis::atLeft);
if (yAxis != nullptr) {
yAxisList.append(yAxis);
}
}
```
2. 遍历每个y轴对应的曲线,获取最小值和最大值。
```cpp
double minY = std::numeric_limits<double>::max();
double maxY = std::numeric_limits<double>::min();
for (int i = 0; i < yAxisList.length(); i++) {
QCPAxis *yAxis = yAxisList[i];
minY = std::numeric_limits<double>::max();
maxY = std::numeric_limits<double>::min();
for (int j = 0; j < ui->customPlot->graphCount(); j++) {
QCPGraph *graph = ui->customPlot->graph(j);
if (graph->dataCount() == 0) {
continue;
}
if (graph->valueAxis() != yAxis) {
continue;
}
QCPGraphDataContainer::const_iterator it = graph->data().constBegin();
for (; it != graph->data().constEnd(); ++it) {
double value = it->value;
if (value < minY) {
minY = value;
}
if (value > maxY) {
maxY = value;
}
}
}
}
```
3. 计算自适应的范围,并设置每个y轴的范围。
```cpp
double range = qMax(qAbs(minY), qAbs(maxY)) * 2;
double center = (minY + maxY) / 2;
for (int i = 0; i < yAxisList.length(); i++) {
QCPAxis *yAxis = yAxisList[i];
yAxis->setRange(center - range, center + range);
}
```
完整代码示例:
```cpp
QList<QCPAxis*> yAxisList;
for (int i = 0; i < ui->customPlot->axisRectCount(); i++) {
QCPAxisRect *axisRect = ui->customPlot->axisRect(i);
QCPAxis *yAxis = axisRect->axis(QCPAxis::atLeft);
if (yAxis != nullptr) {
yAxisList.append(yAxis);
}
}
for (int i = 0; i < yAxisList.length(); i++) {
QCPAxis *yAxis = yAxisList[i];
double minY = std::numeric_limits<double>::max();
double maxY = std::numeric_limits<double>::min();
for (int j = 0; j < ui->customPlot->graphCount(); j++) {
QCPGraph *graph = ui->customPlot->graph(j);
if (graph->dataCount() == 0) {
continue;
}
if (graph->valueAxis() != yAxis) {
continue;
}
QCPGraphDataContainer::const_iterator it = graph->data().constBegin();
for (; it != graph->data().constEnd(); ++it) {
double value = it->value;
if (value < minY) {
minY = value;
}
if (value > maxY) {
maxY = value;
}
}
}
double range = qMax(qAbs(minY), qAbs(maxY)) * 2;
double center = (minY + maxY) / 2;
yAxis->setRange(center - range, center + range);
}
```
ui->customPlot->lockAxisY(2);此接口不存在
看起来您提到的`ui->customPlot->lockAxisY(2)`可能是误记或者某个特定环境下的自定义功能,因为在QCustomPlot库(这是一个Qt库中的绘图组件)中并没有直接提供名为`lockAxisY`的函数。QCustomPlot中的确允许开发者通过API锁定特定轴,例如防止自动缩放,但这通常通过其他方法实现,比如使用`setFixedScale`、`setAxisEnabled`等函数来分别控制轴是否可以动态调整范围。
如果你想要锁定某个轴,可能需要查阅具体的文档或者查找相关的示例代码,找出替代的方式来实现类似的功能。例如,你可以尝试:
```cpp
ui->customPlot->axisRect()->enableAxis(QCPAxis::yBottom, false); // 关闭Y轴下限的自动调整
```
或者
```cpp
ui->customPlot->yAxis->setAutoRangeMode(QCPAxis::Range::ZeroToUser); // 设置Y轴范围仅限于用户设定
```
然而,如果没有官方提供的相应函数,就需要你自己根据需求编写额外的逻辑来维护轴的状态。
阅读全文
相关推荐
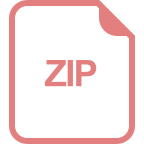
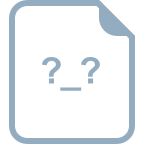
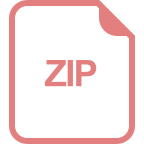











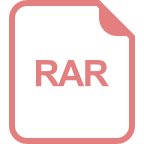
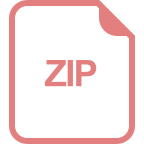