C# EF 5.0通用类,请封装实现给出源码
时间: 2024-10-24 08:08:16 浏览: 27
C# EF (Entity Framework) 是 Microsoft 提供的一个用于.NET平台的对象关系映射工具,它允许开发者将数据库操作转换为对对象的操作。EF 5.0 中的通用类通常是指那些可以复用的实体框架辅助类,例如Repository Pattern(仓储模式)的实现,用于数据访问层的设计。
下面是一个简单的Repository通用类的示例,这个例子中,` EfGenericRepository<T>` 是一个泛型类,`T` 是你想操作的数据上下文类型(如 `DbContext` 或其派生类),`MyDbContext` 是具体的数据库上下文类:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
public class EfGenericRepository<T> where T : DbContext
{
private readonly T _dbContext;
public EfGenericRepository(T dbContext)
{
_dbContext = dbContext;
}
// CRUD操作示例
public List<TEntity> GetAll<TEntity>() where TEntity : class
{
return _dbContext.Set<TEntity>().ToList();
}
public TEntity GetById<TEntity>(object id) where TEntity : class
{
return _dbContext.Set<TEntity>().Find(id);
}
public void Add(T entity)
{
_dbContext.Set(entity.GetType()).Add(entity);
}
public void Update(T entity)
{
_dbContext.Entry(entity).State = EntityState.Modified;
}
public void Delete(T entity)
{
_dbContext.Set(entity.GetType()).Remove(entity);
}
public int SaveChanges()
{
return _dbContext.SaveChanges();
}
}
// 使用示例
public class MyDbContext : DbContext
{
public DbSet<MyEntity> MyEntities { get; set; }
}
public class MyClassService
{
private readonly EfGenericRepository<MyDbContext> _repository;
public MyClassService(EfGenericRepository<MyDbContext> repository)
{
_repository = repository;
}
public List<MyEntity> GetAllEntities()
{
return _repository.GetAll<MyEntity>();
}
}
```
阅读全文
相关推荐
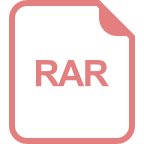
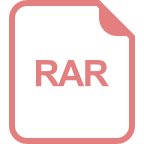
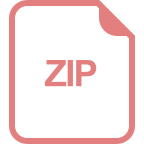
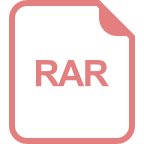
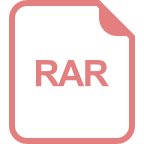
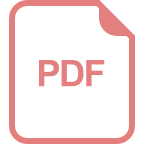
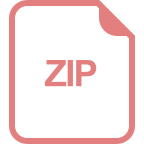
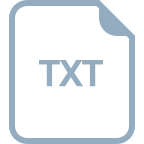
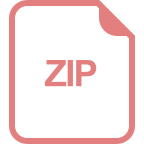
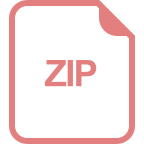
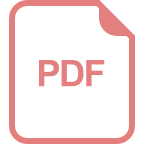
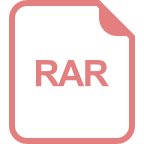
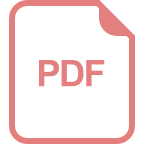
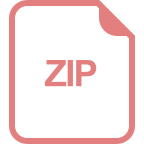