用c语言编制程序,利用凯撒密码,对下面的文字进行加密:“I like the course of information security"。其中的空格可用字母'x'来代替。并尝试利用解密算法对密文进行解密。
时间: 2024-09-25 19:05:32 浏览: 37
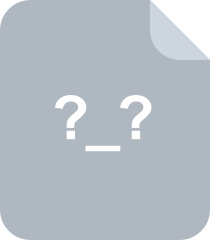
凯撒密码c语言 凯撒密码c语言
凯撒密码是一种简单的替换式加密技术,它通过将明文中的每个字符向后移动固定位数来进行加密。在C语言中,我们可以编写一个函数来完成这个过程。这里我们假设位移量为3(例如常见的 Caesar Cipher)。首先,加密函数会将字母表中的字符向前移动指定位置,如果超过'A'则回到字母表的开头。对于空格和非字母字符,通常不变。
```c
#include <stdio.h>
#include <string.h>
char shift(char c, int shift_amount) {
if (c >= 'A' && c <= 'Z') {
return ((c - 'A' + shift_amount) % 26) + 'A';
} else if (c >= 'a' && c <= 'z') {
return ((c - 'a' + shift_amount) % 26) + 'a';
} else {
return c;
}
}
void caesar_encrypt(char *plaintext, char *ciphertext, int shift_amount) {
for (int i = 0; plaintext[i] != '\0'; i++) {
ciphertext[i] = shift(plaintext[i], shift_amount);
// 对于空格,用'x'代替
if (plaintext[i] == ' ') {
ciphertext[i] = 'x';
}
}
ciphertext[i] = '\0'; // 确保字符串结束
}
// 解密函数只需将shift_amount取负即可
void caesar_decrypt(char *ciphertext, char *plaintext, int shift_amount) {
shift_amount *= -1;
for (int i = 0; ciphertext[i] != '\0'; i++) {
plaintext[i] = shift(ciphertext[i], shift_amount);
// 如果原本是'x',还原为空格
if (ciphertext[i] == 'x') {
plaintext[i] = ' ';
}
}
plaintext[i] = '\0';
}
int main() {
char original[] = "I like the course of information security";
char encrypted[50]; // 假设文本最长不超过49个字符
char decrypted[50];
caesar_encrypt(original, encrypted, 3);
printf("Encrypted text: %s\n", encrypted);
caesar_decrypt(encrypted, decrypted, 3);
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
运行这个程序,你会得到加密后的密文以及解密后的原文。注意,由于凯撒密码的简单性,它并不是非常安全的加密手段,适用于简单的教学演示。
阅读全文
相关推荐
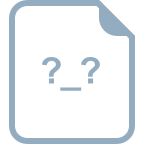
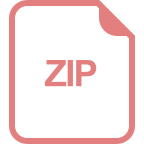
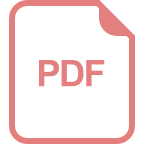
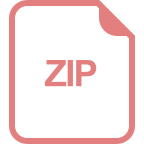
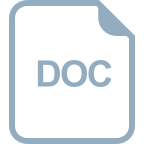
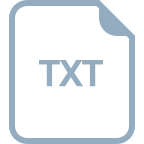
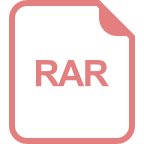
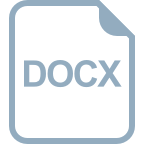
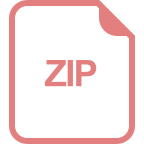
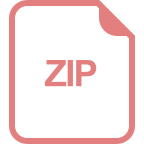
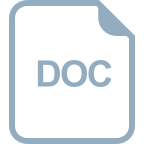