父组件调用子组件初始化方法
时间: 2024-01-07 13:22:22 浏览: 39
在Vue中,父组件调用子组件的初始化方法可以通过以下几种方式实现:
1. 使用`ref`属性和`$refs`对象:
```vue
<template>
<div>
<child-component ref="childRef"></child-component>
<button @click="callChildMethod">调用子组件初始化方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
callChildMethod() {
this.$refs.childRef.init();
}
}
};
</script>
```
2. 使用`$children`属性:
```vue
<template>
<div>
<child-component></child-component>
<button @click="callChildMethod">调用子组件初始化方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
callChildMethod() {
const childComponent = this.$children.find(child => child.$options.name === 'ChildComponent');
if (childComponent) {
childComponent.init();
}
}
}
};
</script>
```
3. 使用事件机制:
父组件通过`$emit`方法触发一个自定义事件,子组件监听该事件并执行初始化方法。
```vue
// 父组件
<template>
<div>
<child-component @init="callChildMethod"></child-component>
<button @click="callChildMethod">调用子组件初始化方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
callChildMethod() {
this.$emit('initChild');
},
childInit() {
console.log('子组件初始化方法');
}
}
};
</script>
// 子组件
<template>
<div>
子组件内容
</div>
</template>
<script>
export default {
mounted() {
this.$parent.$on('initChild', this.init);
},
methods: {
init() {
console.log('子组件初始化方法');
}
}
};
</script>
```
相关推荐
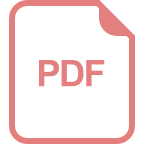
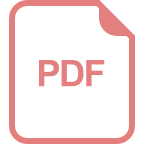
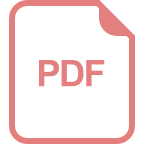














