如何在C++中遍历并打印一个字符串数组中的每个字符的ASCII码,并同时找出并输出最大ASCII值?
时间: 2024-12-12 11:26:10 浏览: 8
在C++中,你可以通过迭代器或者直接下标来遍历字符串数组中的每个字符,然后使用`char`类型的内置函数`ord()`或`static_cast<int>(ch)`来获取ASCII码。这里是一个简单的例子:
```cpp
#include <iostream>
#include <cctype> // 包含ord()函数
// 函数模板用于遍历数组并找到最大ASCII值
template<typename T>
int findMaxASCII(const T* arr, int size) {
int maxASCII = arr[0];
for (int i = 1; i < size; ++i) {
if (arr[i] > maxASCII) {
maxASCII = arr[i];
}
}
return maxASCII;
}
int main() {
std::string strArray[] = {"Hello, ASCII World!"};
const int strSize = sizeof(strArray) / sizeof(strArray[0]);
// 遍历并打印每个字符及其ASCII码
for (const auto& ch : strArray) {
int ascii = static_cast<int>(ch);
std::cout << "Character: " << ch << ", ASCII Code: " << ascii << std::endl;
}
// 找出并打印最大ASCII值
int maxASCII = findMaxASCII(&strArray[0], strSize);
std::cout << "Max ASCII value: " << maxASCII << std::endl;
return 0;
}
```
在这个程序中,我们首先创建了一个字符串数组,然后使用范围for循环遍历数组中的每个字符,并将其转换为ASCII码打印出来。接着,我们调用`findMaxASCII`函数来查找整个数组中最大的ASCII值。
阅读全文
相关推荐
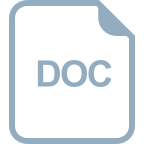
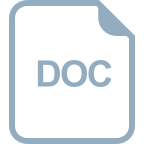
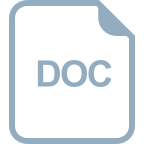
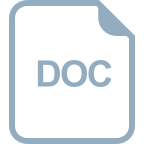
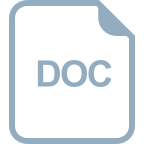













