c++中如何让对种群内个体进行排序排序
时间: 2024-09-10 07:20:58 浏览: 35
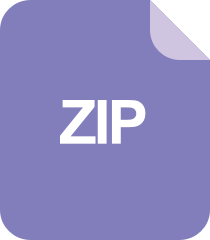
高分项目,基于C++开发实现的带精英策略的非支配排序遗传算法(NSGA-II),内含完整源码+PPT文档
在C++中,对种群内的个体进行排序通常涉及到使用标准模板库(STL)中的排序函数。这里以`std::sort`函数为例,展示如何进行排序。假设我们有一个种群类`Population`,其中包含一个个体数组或者向量`individuals`,每个个体都有一个可以比较的属性,比如适应度(fitness)。
首先,你需要定义个体类并为其实现比较运算符,或者定义比较函数。以下是简单的示例代码:
```cpp
#include <algorithm> // 引入算法库
// 定义个体类
class Individual {
public:
// 构造函数
Individual(int fit) : fitness(fit) {}
// 适应度属性
int fitness;
// 重载小于运算符,用于排序
bool operator<(const Individual& other) const {
return fitness < other.fitness; // 按适应度升序排列
}
};
// 定义种群类
class Population {
public:
std::vector<Individual> individuals;
// 对种群内的个体进行排序的方法
void sortIndividuals() {
std::sort(individuals.begin(), individuals.end());
}
};
// 使用示例
int main() {
Population population;
// 假设种群初始化完毕
// 对种群中的个体进行排序
population.sortIndividuals();
// 排序后的种群
// ...
return 0;
}
```
在上述代码中,`std::sort`默认按照升序对元素进行排序。如果你需要按照其他标准(如降序)进行排序,可以传递第三个参数来指定比较函数或者lambda表达式。
阅读全文
相关推荐
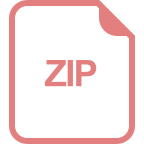
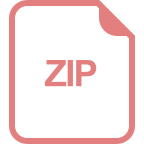

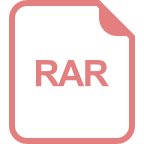
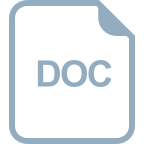
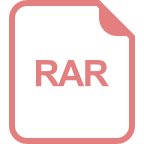
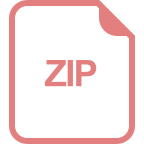
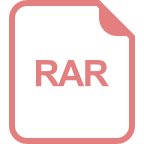
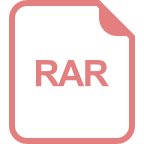
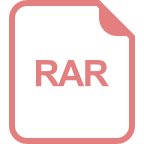
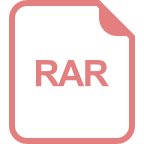
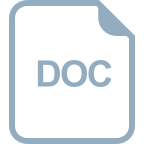
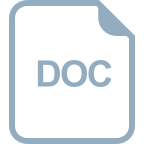




