用数据结构写出复数的主函数
时间: 2024-11-08 11:12:29 浏览: 16
复数可以使用两个整数分别表示实部和虚部来表示,通常我们会选择将它们封装在一个自定义的数据结构中,比如在C++中可以创建一个名为`Complex`的结构体或者类。下面是一个简单的`Complex`类的例子,包含构造函数、赋值运算符等基本操作:
```cpp
#include <iostream>
// 定义复数类 Complex
class Complex {
private:
int real; // 实部
int imag; // 虚部
public:
// 构造函数,初始化复数
Complex(int r = 0, int i = 0) : real(r), imag(i) {}
// 获取实部和虚部
int getReal() const { return real; }
int getImaginary() const { return imag; }
// 设置复数
void set(Complex& other) {
real = other.real;
imag = other.imag;
}
// 加法运算
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 输出复数
friend std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.real << " + " << c.imag << "i";
return os;
}
};
int main() {
Complex a(3, 4); // 创建复数a = 3+4i
Complex b(1, -2); // 创建复数b = 1-2i
std::cout << "a = " << a << std::endl;
std::cout << "b = " << b << std::endl;
// 操作演示
Complex c = a + b; // 相加得到c = 4+2i
std::cout << "c = a + b = " << c << std::endl;
return 0;
}
```
在这个例子中,`main`函数展示了如何创建复数对象并执行一些基础操作,如相加。
阅读全文
相关推荐
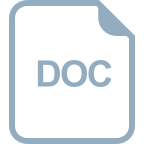
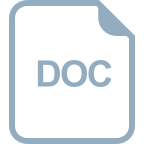
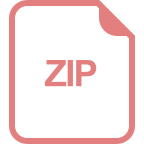
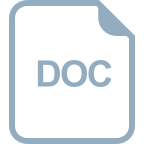
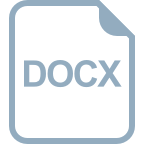
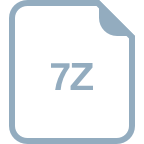
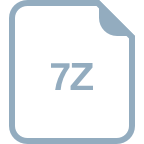
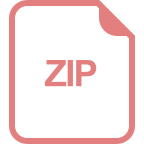
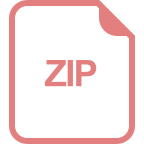
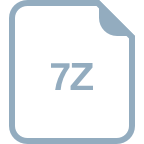
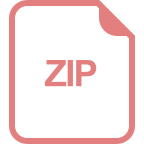