Java 解压zip
时间: 2024-09-28 16:00:28 浏览: 35
在Java中,你可以使用内置库`java.util.zip`来解压`.zip`文件。以下是使用`ZipInputStream`和`ZipEntry`类的基本步骤:
```java
import java.io.*;
import java.util.zip.*;
public class UnzipFile {
public static void main(String[] args) {
try {
// 1. 创建输入流,指定zip文件路径
FileInputStream zipFile = new FileInputStream("path_to_your_zip_file.zip");
// 2. 创建ZipInputStream,读取zip文件
ZipInputStream zin = new ZipInputStream(zipFile);
// 3. 创建一个BufferedInputStream用于缓存数据
BufferedInputStream bin = new BufferedInputStream(zin);
// 4. 使用ZipEntry遍历文件列表
ZipEntry entry;
while ((entry = zin.getNextEntry()) != null) {
// 检查是否是目录
if (!entry.isDirectory()) {
// 5. 创建输出目录和输出流
String filePath = "output/" + entry.getName();
File dir = new File(filePath.substring(0, filePath.lastIndexOf('/')));
if (!dir.exists()) {
dir.mkdirs(); // 如果不存在就创建目录
}
// 6. 创建FileOutputStream写入解压后的文件
FileOutputStream out = new FileOutputStream(filePath);
// 7. 将缓冲的数据写入到输出流
byte[] buffer = new byte[1024];
int length;
while ((length = bin.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
// 8. 关闭流
out.close();
bin.close();
}
zin.closeEntry(); // 结束当前文件处理
}
// 9. 最后关闭所有打开的流
zin.close();
bin.close();
zipFile.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
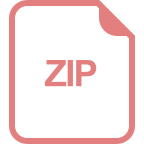
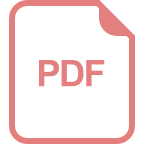
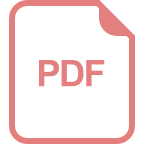
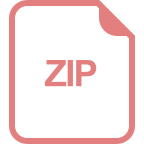
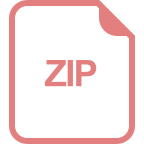
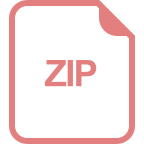
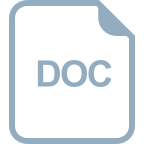
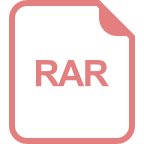
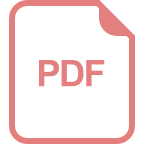
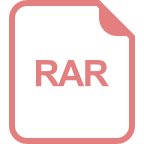
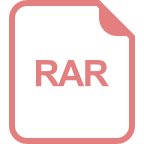
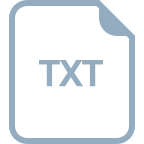
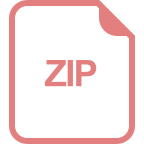
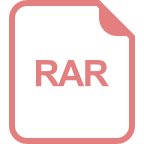

