在idea中实现点击查询按钮是将c表数据删除同时将a,b表数据依次插入,最后将c表数据展示。其mybatis.xml文件,mapper接口文件以及相关的impl文件,service,controller层的文件如何编写,方法有那些详细举例说明,含义
时间: 2024-11-15 17:25:45 浏览: 26
在IntelliJ IDEA中实现上述功能需要创建一系列关联的文件。这里我会简单描述每个层级的文件结构和关键方法的实现。
1. **mybatis.xml** (Mapper XML文件)
```xml
<sql id="insertSqls">
INSERT INTO a (field_a) VALUES (:valueA);
INSERT INTO b (field_b) VALUES (:valueB);
</sql>
<update id="deleteAndUpdateC">
DELETE FROM c WHERE condition;
<foreach item="item" index="index" collection="list" open="(" separator="," close=")">
INSERT INTO c (field_c) VALUES (#{item.value});
</foreach>
</update>
<select id="selectAllC" resultType="com.example.CEntity">
SELECT * FROM c;
</select>
```
2. **Mapper Interface** (Mapper接口文件)
```java
public interface CMapper {
int insertAB(List<Map<String, Object>> mapList);
int deleteAndUpdateC();
List<CEntity> selectAllC();
}
```
3. **Mapper Impl** (Mapper Implementation文件)
```java
public class CMapperImpl implements CMapper {
private final SqlSession sqlSession;
public CMapperImpl(SqlSession sqlSession) {
this.sqlSession = sqlSession;
}
@Override
public int insertAB(List<Map<String, Object>> mapList) {
return sqlSession.insert("insertSqls", mapList);
}
@Override
public int deleteAndUpdateC() {
return sqlSession.update("deleteAndUpdateC");
}
@Override
public List<CEntity> selectAllC() {
return sqlSession.selectList("selectAllC");
}
}
```
4. **Service Layer** (Service类)
```java
@Service
public class CService {
private final CMapper mapper;
public CService(CMapper mapper) {
this.mapper = mapper;
}
public void clickQueryButton(List<Map<String, Object>> data) {
// 删除C表数据并插入A、B表数据
int result = mapper.deleteAndUpdateC();
// 如果删除成功,获取更新后的C表数据并显示
if (result > 0) {
List<CEntity> entities = mapper.selectAllC();
// 处理数据并展示
for (CEntity entity : entities) {
// 打印或存入UI
System.out.println(entity);
}
}
}
}
```
5. **Controller Layer** (Controller类)
```java
@RestController
@RequestMapping("/api/c")
public class CController {
@Autowired
private CService service;
@PostMapping("/clickQuery")
public ResponseEntity<?> clickQuery(@RequestBody List<Map<String, Object>> data) {
try {
service.clickQueryButton(data);
return ResponseEntity.ok().build();
} catch (Exception e) {
return ResponseEntity.badRequest().body(e.getMessage());
}
}
}
```
在这个例子中,用户通过POST请求`/api/c/clickQuery`提交数据,服务层`CService`根据这些数据执行删除和插入操作,然后查询并返回结果。注意,具体的字段名和条件应根据实际情况调整,并且异常处理可以根据需要添加更详细的日志或通知。
阅读全文
相关推荐
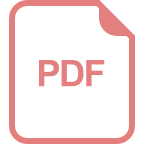
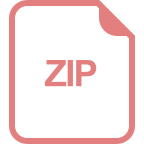
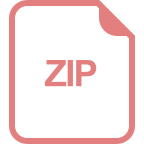
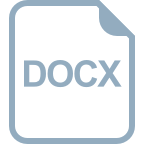
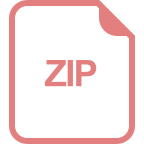

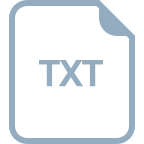
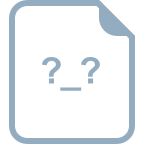
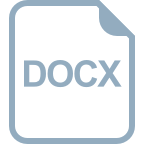
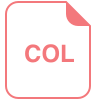
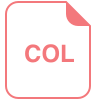
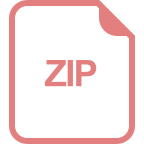