任务:给定一个字符串,该字符串的任务:给定一个字符串,该字符串的内容为一个英语短句,请编写代码实现单词的反向排序。 """内容为一个英语短句,请编写代码实现单词的反向排序。 """
时间: 2024-12-10 10:31:40 浏览: 9
为了实现这个功能,你可以按照以下步骤编写 Python 代码:
```python
def reverse_words_in_sentence(sentence):
# 将句子拆分成单词列表
words = sentence.split()
# 反转单词列表
reversed_words = words[::-1]
# 重新组合单词为反向排列的句子,用空格分隔
reversed_sentence = ' '.join(reversed_words)
return reversed_sentence
# 示例输入
sentence = "Hello World, this is a test"
# 调用函数并打印结果
reversed_sentence = reverse_words_in_sentence(sentence)
print("原句:", sentence)
print("反向排序后的句子:", reversed_sentence)
```
在这个代码中,我们首先使用 `split()` 函数将句子分割成单词列表,然后通过切片操作 `[::1]` 反转列表,最后使用 `join()` 函数将单词重新连接成字符串。
相关问题
Python练习字符串答案头歌
Python中有一个有趣的习题,涉及到字符串操作,可以创建一个“头歌”效果,即把一个给定的句子首字母大写的每个单词形成一个新的短句。这个过程通常被称为“标题化”(Title Case)。例如,输入"hello world"会变成"HeLlO wOrLd"。
下面是一个简单的Python函数示例,用于实现这个功能:
```python
def title_song(sentence):
# 使用内置的split()函数将句子拆分成单词列表
words = sentence.split()
# 对每个单词的首字母转换为大写,其余小写,然后连接成新的句子
title_words = [word.title() for word in words]
# 用空格连接所有的单词形成新句子
title_song_str = ' '.join(title_words)
return title_song_str
# 测试函数
sentence = "hello world"
print(title_song(sentence)) # 输出:HeLlO wOrLd
```
阅读全文
相关推荐
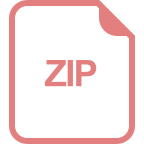
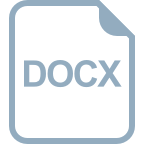
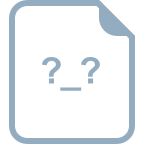
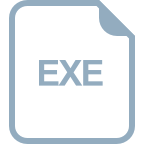
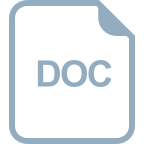
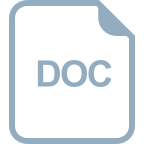
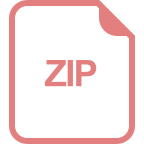