java 上传文件到Windows服务器
时间: 2024-10-18 10:16:46 浏览: 29
在Java中,上传文件到Windows服务器通常使用HTTP或FTP协议,这里以HTTP为例,因为HTTP是最常见的Web服务协议,许多服务器都支持它。以下是一个简单的示例,使用`HttpURLConnection`来上传文件:
1. 首先,创建一个`URL`对象,指明服务器的URL加上文件上传的端点,例如`/upload`:
```java
URL uploadUrl = new URL("http://your_server.com/upload");
```
替换 `your_server.com` 为你的Windows服务器的实际域名或IP地址。
2. 使用`HttpURLConnection`实例化,并设置请求方法为POST,同时设置允许输入:
```java
HttpURLConnection conn = (HttpURLConnection) uploadUrl.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
```
3. 设置请求头,特别是Content-Type(表明你要上传的是二进制文件)和Authorization(如果需要认证):
```java
String auth = "Basic " + Base64.getEncoder().encodeToString(("username:password").getBytes());
conn.setRequestProperty("Authorization", auth);
conn.setRequestProperty("Content-Type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
```
替换 `username` 和 `password` 为你服务器的登录凭据。
4. 获取输出流,准备发送文件:
```java
DataOutputStream wr = new DataOutputStream(conn.getOutputStream());
```
5. 构建边界线和文件内容的数据分段:
```java
StringBuilder content = new StringBuilder();
content.append("--").append("----WebKitFormBoundary7MA4YWxkTrZu0gW\r\n")
.append("Content-Disposition: form-data; name=\"file\"; filename=\"" + file.getName() + "\"\r\n")
.append("Content-Type: application/octet-stream\r\n\r\n");
// 写入文件内容
byte[] bytes = Files.readAllBytes(file.toPath());
content.append(new String(bytes)).append("\r\n");
content.append("--").append("----WebKitFormBoundary7MA4YWxkTrZu0gW--\r\n");
wr.write(content.toString().getBytes());
```
这里的`file`是你要上传的本地文件的`File`对象。
6. 关闭流并检查响应状态码:
```java
wr.flush();
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("Upload successful");
} else {
System.err.println("Upload failed: " + responseCode);
}
wr.close();
conn.disconnect();
```
阅读全文
相关推荐
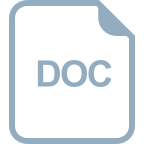
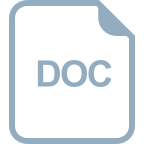
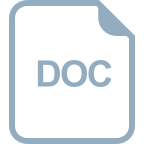
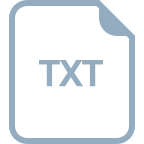
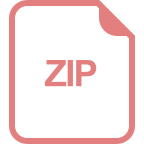



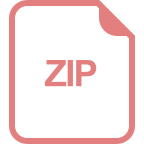
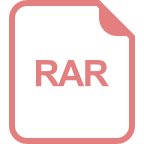
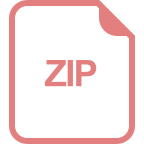
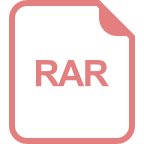





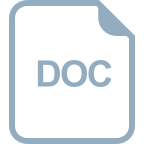
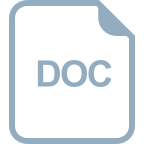