给出一个python的例子,在输入的字符串中去掉回车符号
时间: 2024-09-28 21:03:31 浏览: 31
在Python中,你可以使用`replace()`函数或者切片操作来去除字符串中的回车符(`\n`)。这里是一个例子:
```python
def remove_carriage_return(s):
return s.replace('\n', '')
# 或者使用切片
def remove_carriage_return_short(s):
return s.rstrip()
input_string = "Hello\nWorld!"
cleaned_string = remove_carriage_return(input_string)
print(cleaned_string)
# 使用短版本
short_cleaned_string = remove_carriage_return_short(input_string)
print(short_cleaned_string)
```
在这个例子中,`remove_carriage_return`函数会将所有的`\n`替换为空字符串,而`remove_carriage_return_short`则只移除字符串结尾的回车。
相关问题
给出python例子,在一段字符串中,匹配不带目录的py文件名
在Python中,你可以使用正则表达式(`re`模块)来匹配一个字符串中的不带目录的.py文件名。下面是一个简单的例子:
```python
import re
def match_python_files(string):
pattern = r'\b(?<!\/)[a-zA-Z0-9_\.]+\.py\b'
matches = re.findall(pattern, string)
return matches
# 示例字符串
example_string = "my_project/subdir/file1.py, another_file.py, not_a_file.txt"
# 匹配并打印结果
matches = match_python_files(example_string)
print("Matched Python file names:", matches)
```
在这个例子中,`pattern`正则表达式的含义是:
- `\b`: 单词边界,避免匹配包含完整路径的部分
- `(?<!\/)`: 使用否定前瞻断言,确保前面不是斜杠 `/`,防止误匹配路径
- `[a-zA-Z0-9_\.]+`: 匹配字母、数字、下划线或点字符组成的一组,这通常对应于文件名部分
- `.py`: 直接匹配`.py`,表示文件扩展名为.py
python 查询一个字符串在另一个字符串中的位置
在Python中,你可以使用内置函数`str.index()`或者`str.find()`来查询一个字符串在另一个字符串中的位置。这两种方法都会返回子字符串第一次出现的位置索引,如果子字符串不存在于主字符串中,则会抛出异常。
1. `index()`方法:
```python
main_string = "Hello, Python!"
sub_string = "Python"
position = main_string.index(sub_string)
print(position) # 输出: 7 (从0开始计数)
```
2. `find()`方法:
```python
main_string = "Hello, Python!"
sub_string = "Python"
position = main_string.find(sub_string)
if position != -1:
print(position) # 输出: 7
else:
print("子字符串未找到")
```
注意,`find()`方法如果找不到子字符串,会返回-1。如果你想处理找不到的情况,可以使用条件判断。
阅读全文
相关推荐
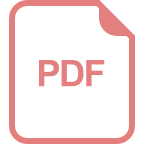
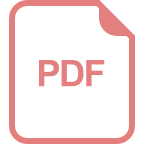
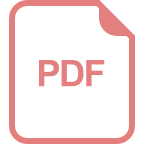













