使用HBase java api 创建名为‘Patients’的HBase表,并导入数据集patients.csv,导入前先创建列簇“demographics”‘anthropometry’‘medical-history’及列限定符
时间: 2024-10-12 10:05:59 浏览: 86
在使用HBase Java API创建名为`Patients`的表并导入CSV数据之前,你需要做以下几个步骤:
1. **设置环境**:
首先,确保你已经添加了HBase的Java客户端依赖到项目中,并且设置了HBase的配置。
2. **创建表**:
使用`HBaseAdmin`类创建表,指定表名`Patients`,以及列簇(Column Families)。例如:
```java
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.util.Bytes;
public void createTable() {
HBaseConfiguration config = HBaseConfiguration.create();
Admin admin = new Admin(config);
TableName tableName = TableName.valueOf("Patients");
byte[] demographicCF = Bytes.toBytes("demographics");
byte[] anthropometryCF = Bytes.toBytes("anthropometry");
byte[] medicalHistoryCF = Bytes.toBytes("medical-history");
// 定义列簇信息
HBaseTableDescriptor tableDesc = new HBaseTableDescriptor(tableName);
ColumnFamilyDescriptor demographicCFDesc = new ColumnFamilyDescriptor(demographicCF);
ColumnFamilyDescriptor anthropometryCFDesc = new ColumnFamilyDescriptor(anthropometryCF);
ColumnFamilyDescriptor medicalHistoryCFDesc = new ColumnFamilyDescriptor(medicalHistoryCF);
// 添加列簇到表描述
tableDesc.addFamily(demographicCFDesc);
tableDesc.addFamily(anthropometryCFDesc);
tableDesc.addFamily(medicalHistoryCFDesc);
try {
admin.createTable(tableDesc);
System.out.println("Table " + tableName.getNameAsString() + " created successfully.");
} catch (IOException e) {
System.err.println("Error creating table: " + e.getMessage());
}
admin.close();
}
```
3. **导入数据**:
对于导入CSV文件,可以使用`HFileLoader`或者第三方库如`Hadoop Streaming`或者`HBase Client`提供的`loadIncrementalHFiles`方法。这里需要将CSV中的每一行解析为HBase Put操作,然后批量写入。由于这涉及到文件处理和复杂的数据转换,代码会比较长,所以通常建议使用适当的工具包如Apache Commons CSV等辅助。
4. **导入前的准备**:
在导入数据前,确认CSV文件的第一行是列标题,且格式对应HBase列族和列限定符的结构。例如:
```csv
PatientID,demographics:age,demographics:gender,anthropometry:height,anthropometry:weight,medical-history:diabetes
```
然后在Java代码中读取这个文件并将其拆分成Put操作。
5. **数据加载示例(简化版)**:
```java
List<Put> puts = new ArrayList<>();
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader("patients.csv"));
String line;
while ((line = reader.readLine()) != null) {
// 解析CSV行并构建Put操作
Put put = new Put(Bytes.toBytes(line.split(",", -1)[0]));
for (String field : line.split(",")) {
int colonIndex = field.indexOf(':');
if (colonIndex > 0) {
byte[] columnQualifier = Bytes.toBytes(field.substring(colonIndex + 1));
put.addColumn(Bytes.toBytes(field.substring(0, colonIndex)), columnQualifier, Bytes.toBytes(field.substring(colonIndex + 2)));
}
}
puts.add(put);
}
} finally {
if (reader != null) {
try { reader.close(); } catch (IOException e) {}
}
}
try {
admin.loadIncrementalHFiles(puts.stream().map(Put::getRow).collect(Collectors.toList()));
System.out.println("Data imported successfully.");
} catch (IOException e) {
System.err.println("Error importing data: " + e.getMessage());
}
```
记得在实际应用中处理异常和日志。
阅读全文
相关推荐
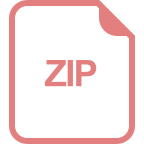
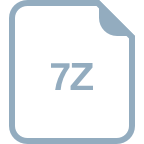
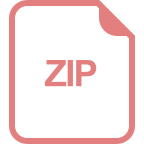

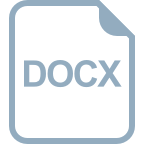
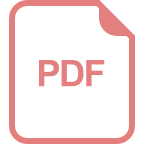
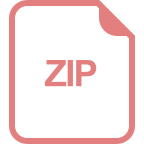
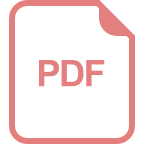
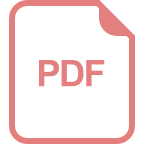
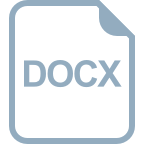
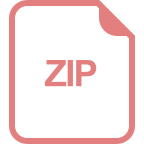
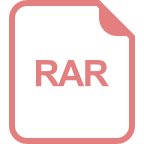
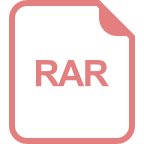
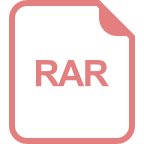
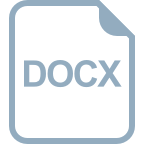
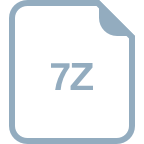
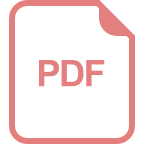