用c#语言设计开发一个控制台应用程序,实现两个数的加减乘除运算; 2、加减乘除分别由四个静态方法实现,由主方法调用完成功能。
时间: 2024-10-29 20:11:48 浏览: 49
在C#中,你可以创建一个控制台应用程序,通过定义一个类并为每个基本数学运算设置静态方法来实现需求。这里有一个简单示例:
```csharp
using System;
public class MathOperations
{
// 加法运算
public static double Add(double num1, double num2)
{
return num1 + num2;
}
// 减法运算
public static double Subtract(double num1, double num2)
{
return num1 - num2;
}
// 乘法运算
public static double Multiply(double num1, double num2)
{
return num1 * num2;
}
// 除法运算(包含错误处理)
public static double Divide(double num1, double num2)
{
if (num2 != 0)
return num1 / num2;
else
throw new ArgumentException("Cannot divide by zero.");
}
}
class Program
{
static void Main(string[] args)
{
Console.Write("Enter the first number: ");
double num1 = Convert.ToDouble(Console.ReadLine());
Console.Write("Enter the second number: ");
double num2 = Convert.ToDouble(Console.ReadLine());
try
{
// 调用静态方法
Console.WriteLine($"Addition: {MathOperations.Add(num1, num2)}");
Console.WriteLine($"Subtraction: {MathOperations.Subtract(num1, num2)}");
Console.WriteLine($"Multiplication: {MathOperations.Multiply(num1, num2)}");
Console.WriteLine("Enter a number to perform division (or press enter for an exception): ");
string input = Console.ReadLine();
if (!string.IsNullOrEmpty(input))
Console.WriteLine($"Division: {MathOperations.Divide(num1, Double.Parse(input))}");
else
Console.WriteLine("No division performed due to user input.");
}
catch (FormatException e)
{
Console.WriteLine($"Invalid format: {e.Message}");
}
catch (ArgumentException ex)
{
Console.WriteLine(ex.Message);
}
Console.ReadKey();
}
}
```
这个程序首先提示用户输入两个数字,然后调用 `MathOperations` 类中的静态方法进行加减乘运算,并在尝试除法运算之前询问用户是否继续。如果用户输入了非数字,会捕获 `FormatException`;若用户试图除以零,会捕获 `ArgumentException`。
阅读全文
相关推荐


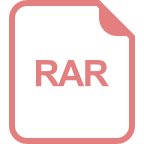

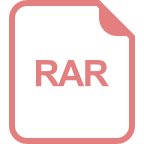
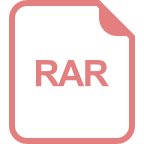
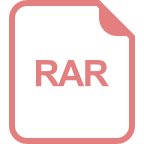
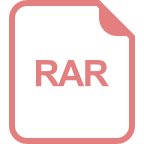










