图片上传 回显 uniapp
时间: 2024-12-27 11:23:35 浏览: 4
### 实现 UniApp 图片上传并回显预览
在 UniApp 中实现图片上传后的回显功能涉及前端页面设计和 JavaScript 处理逻辑。具体来说,HTML 部分用于构建界面元素,JavaScript 负责处理文件读取、上传请求及更新视图状态。
#### HTML 部分
创建一个按钮触发文件选择器,并预留位置显示已选中的图片缩略图:
```html
<template>
<view class="upload-section">
<!-- 文件输入框 -->
<button type="primary" @click="chooseImage">选择图片</button>
<!-- 已选图片展示区 -->
<block v-for="(item, index) in imageList" :key="index">
<image :src="item.url" mode="aspectFit"></image>
<text>{{ item.name }}</text>
<icon type="clear" size="18" color="#ff0000" @tap="removeImage(index)" />
</block>
</view>
</template>
```
#### JavaScript 部分
定义 `data` 属性存储图片列表;编写方法来选取本地图片、执行实际的网络上传操作并将返回的结果保存到组件数据中以便渲染:
```javascript
export default {
data() {
return {
imageList: [] // 存储图片对象数组
};
},
methods: {
chooseImage() {
uni.chooseImage({
count: 9 - this.imageList.length,
success: function(res) {
res.tempFilePaths.forEach((path, idx) => {
let tempObj = {};
tempObj['url'] = path;
tempObj['name'] = '图片' + (this.imageList.length + idx + 1);
// 将临时路径加入待上传队列
this.imageList.push(tempObj);
// 执行上传动作
uploadFilePromise(path).then(response => {
console.log('Upload Success:', response);
// 更新对应项的实际 URL 地址
Object.assign(this.imageList[this.imageList.length - 1], {realUrl: response});
}).catch(error => {
console.error('Failed to Upload', error);
});
}.bind(this));
}.bind(this),
fail(err){
console.warn(`Choose Image Failed:${JSON.stringify(err)}`);
}
});
},
removeImage(idx) {
this.imageList.splice(idx, 1); // 移除指定索引处的对象
}
/**
* 模拟异步上传函数
*/
async function uploadFilePromise(filePath) {
try {
const result = await new Promise(resolve => setTimeout(() => resolve('/static/mock-uploaded-image-url'), 2000)); // 假设这是服务器响应的真实地址
return result;
} catch(e) {
throw e;
}
}
}
}
```
上述代码片段展示了如何通过调用 `uni.chooseImage()` 方法获取用户选定的照片,并将其添加至界面上供查看[^1]。当每张照片被成功上传后,会利用模拟的 `uploadFilePromise()` 函数接收来自服务端反馈的新链接替换原来的临时路径[^2]。
#### CSS 部分
为了使布局更加美观合理,可以适当调整样式表以适应不同尺寸屏幕下的视觉效果:
```css
.upload-section {
padding: 20rpx;
}
.upload-section button {
margin-bottom: 20rpx;
}
.upload-section block {
position: relative;
display: inline-block;
width: 30%;
height: auto;
margin-right: 5%;
vertical-align: top;
}
.upload-section image {
max-width: 100%;
border-radius: 8rpx;
}
.upload-section text {
font-size: 24rpx;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
.upload-section icon {
position: absolute;
top: 0;
right: 0;
background-color: rgba(0, 0, 0, .5);
border-radius: 50%;
line-height: 36rpx;
text-align: center;
}
```
阅读全文
相关推荐
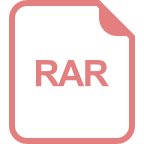
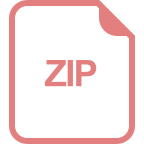
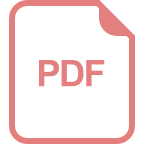















