你好,写个python 五子棋
时间: 2024-11-06 14:12:54 浏览: 7
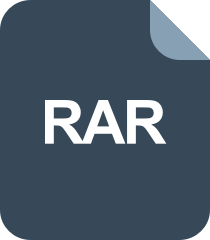
python五子棋项目源码.rar

你好!编写一个简单的Python五子棋游戏,我们可以分为以下几个步骤:
1. 定义棋盘和初始状态
2. 创建用户和电脑玩家的轮流走棋逻辑
3. 判断胜负或平局
4. 用户界面(命令行或图形化)
以下是一个基本的文本模式五子棋游戏的简化代码示例:
```python
import random
class GobangBoard:
def __init__(self, size=15):
self.board = [[' ' for _ in range(size)] for _ in range(size)]
self.size = size
self.current_player = 'X'
def print_board(self):
for row in self.board:
print(' '.join(row))
def place_stone(self, x, y):
if self.board[x][y] != ' ' or (x, y) in self.winning_positions():
return False
self.board[x][y] = self.current_player
if self.check_win(x, y):
return True
self.current_player = 'O' if self.current_player == 'X' else 'X'
return False
def winning_positions(self):
# ...此处省略计算胜利位置的复杂逻辑...
def check_win(self, x, y):
# ...此处省略判断是否五子连珠的逻辑...
def play_game():
board = GobangBoard()
while not board.game_over():
if board.current_player == 'X':
move = int(input("请输入您的步数(x, y): "))
x, y = move // board.size, move % board.size
if board.place_stone(x, y):
print("Player X wins!")
break
else:
random_move = (random.randint(0, board.size - 1),
random.randint(0, board.size - 1))
if board.place_stone(*random_move):
print("Computer wins!")
break
board.print_board()
if __name__ == "__main__":
play_game()
```
这个示例代码非常基础,实际的五子棋游戏会更复杂,包括计算胜利条件、循环直到游戏结束等。如果你想要创建更完整的图形化界面,可能需要使用如Pygame这样的库。
阅读全文
相关推荐
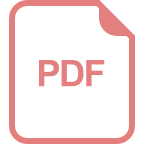
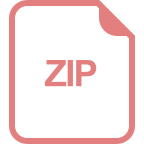
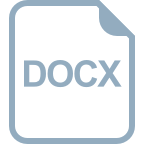
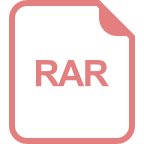
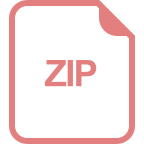
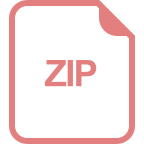
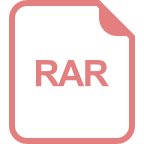
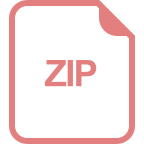
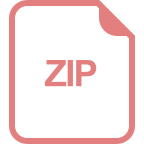
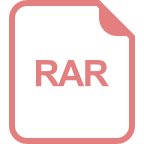
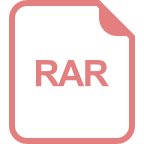
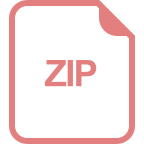