写一个c++程序将ASCII编码下的字符串(无空格)通过二进制转换成Base64编码下的字符串
时间: 2024-10-15 07:26:04 浏览: 40
在C++中,你可以使用标准库函数`std::string`以及一些自定义函数来实现这个功能。下面是一个简单的示例,我们将创建一个名为`ascii_to_base64`的函数,它接受一个ASCII编码的字符串作为输入,然后将其转换为Base64编码:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include "base64.h" // 使用第三方库如`cpp-base64`进行Base64编码
// 将ASCII字符转换为二进制
std::string asciiToBinary(const std::string& asciiStr) {
std::string binary;
for (char c : asciiStr) {
binary += std::to_string(c);
}
return binary;
}
// Base64编码的字符串函数
std::string base64Encode(const std::string& binaryStr) {
std::vector<uint8_t> bytes;
for (int i = 0; i < binaryStr.length(); i += 8) {
uint64_t byte = 0;
for (int j = 0; j < 8 && i + j < binaryStr.length(); ++j) {
byte <<= 1;
if (binaryStr[i + j] == '1') {
byte |= 1;
}
}
bytes.push_back(byte);
}
std::string encoded;
base64::encode(bytes.begin(), bytes.end(), &encoded);
return encoded;
}
int main() {
std::string input("HelloWorld123"); // ASCII字符串示例
std::string binary = asciiToBinary(input);
std::string base64 = base64Encode(binary);
std::cout << "Original ASCII: " << input << "\n";
std::cout << "Binary representation: " << binary << "\n";
std::cout << "Base64 encoded: " << base64 << "\n";
return 0;
}
```
注意:这里我假设你已经有一个名为`cpp-base64`的库来进行Base64编码。实际项目中,你需要安装并引用这个库才能运行上述代码。
阅读全文
相关推荐













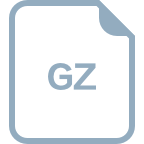


