总共有两个网卡,一个是4G模块,通过usb0连接;另一个以太网,固定到Eth1上面,linux中如何写C语言代码切换4G/以太网
时间: 2024-12-15 14:24:46 浏览: 14
在Linux中,你可以使用`sysfs`目录来动态地管理网络设备,包括切换网络接口。首先,你需要了解`sysfs`是一个虚拟文件系统,允许应用程序读写硬件设备的状态信息。
以下是一个简单的C语言示例,展示了如何打开sysfs路径并切换网络接口。请注意,这只是一个基础示例,并且可能需要根据具体的Linux版本和网络驱动调整:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <string.h>
#define ETH_PATH "/sys/class/net/Eth1"
#define USB_PATH "/sys/class/net/usb0"
// 判断路径是否存在
int path_exists(char *path) {
DIR *dir;
if ((dir = opendir(path)) == NULL)
return 0;
closedir(dir);
return 1;
}
// 设置接口为激活状态
void set_interface_active(const char *interface_path) {
FILE *file;
if ((file = fopen(interface_path "/operstate", "w")) != NULL) {
fprintf(file, "1\n");
fclose(file);
}
}
int main() {
if (path_exists(ETH_PATH)) {
printf("Switching to Ethernet...\n");
set_interface_active(ETH_PATH);
} else if (path_exists(USB_PATH)) {
printf("Switching to 4G module...\n");
set_interface_active(USB_PATH);
} else {
printf("Neither interface found.\n");
return 1;
}
// Add any additional logic or error handling here
return 0;
}
```
这个程序首先检查`Eth1`和`usb0`路径是否存在,然后尝试将相应的接口设置为活跃(即启用)。实际操作中,还需要处理错误情况和确保设备已经正确配置。
阅读全文
相关推荐
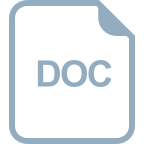
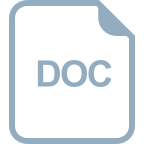
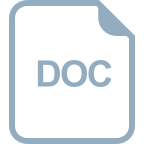

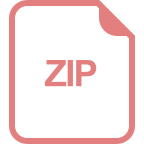
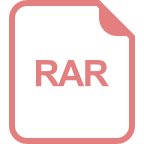
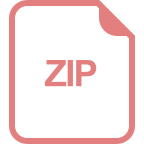
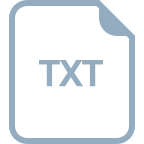
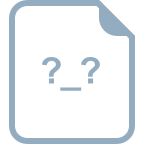
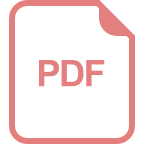
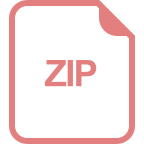
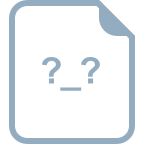
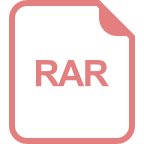
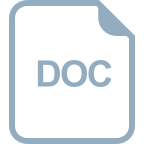
