yolov5 C++
时间: 2023-07-31 12:08:21 浏览: 149
Yolov5 is an object detection algorithm that can be implemented in C++. It is based on the You Only Look Once (YOLO) framework and is the latest version of the YOLO series. To use Yolov5 in C++, you can follow these steps:
1. Install OpenCV: Yolov5 requires OpenCV for image processing. You can download and install OpenCV from the official website or use a package manager like apt-get or Homebrew.
2. Download Yolov5 source code: You can find the Yolov5 source code on the official GitHub repository (https://github.com/ultralytics/yolov5). Clone or download the repository to get the necessary files.
3. Build the C++ project: Navigate to the C++ implementation directory in the Yolov5 source code. Use CMake to generate the build files and compile the project. Make sure to link the required libraries, including OpenCV.
4. Load and run Yolov5: In your C++ code, you need to load the pre-trained Yolov5 model weights and configuration file. Then, you can use the model to perform object detection on images or videos.
Here is a sample code snippet to give you an idea:
```cpp
#include <opencv2/opencv.hpp>
#include "yolov5.h"
int main() {
// Load Yolov5 model
Yolov5 model("yolov5.weights", "yolov5.cfg");
// Load image
cv::Mat image = cv::imread("image.jpg");
// Perform object detection
std::vector<Object> objects = model.detect(image);
// Draw bounding boxes on the image
for (const auto& obj : objects) {
cv::rectangle(image, obj.rect, cv::Scalar(0, 255, 0), 2);
}
// Display the result
cv::imshow("Object Detection", image);
cv::waitKey(0);
return 0;
}
```
Please note that this is just a simplified example, and you may need to modify it based on your specific requirements and the Yolov5 codebase.
阅读全文
相关推荐
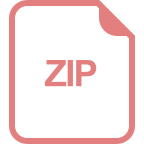










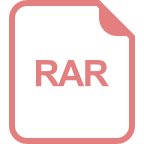
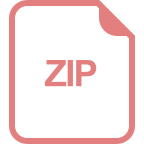
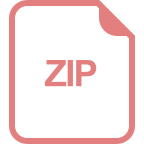