使用c语言,采用链式存储结构,创建一个单链表,实现图书信息的存储,并能完成如下操作 1.建立单链表(头插法或尾插法);2.单链表的输出; 3.单链表的插入操作;4.单链表的删除操作; 5.求单链表的长度;6.取表中第i个元素; 7.在表中査找值为e的元素位置。
时间: 2024-11-03 22:23:36 浏览: 17
在C语言中,我们可以使用链表数据结构来实现图书信息的存储。以下是关于单链表的一些基本操作:
1. **建立单链表** (尾插法):
```c
typedef struct Node {
char title[100]; // 图书标题
int author; // 作者ID
struct Node* next; // 指向下一个节点的指针
} BookNode;
// 尾插法添加节点
void insert(BookNode** head, const char* title, int author) {
BookNode* newNode = (BookNode*)malloc(sizeof(BookNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
strcpy(newNode->title, title);
newNode->author = author;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
BookNode* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
```
2. **单链表的输出**:
```c
void printList(BookNode* head) {
if (head == NULL) {
printf("Empty list.\n");
} else {
BookNode* temp = head;
while (temp != NULL) {
printf("Title: %s, Author: %d\n", temp->title, temp->author);
temp = temp->next;
}
}
}
```
3. **单链表的插入操作**(已在上述`insert`函数中描述)
4. **单链表的删除操作**:
```c
void deleteNode(BookNode** head, int targetAuthor) {
if (*head == NULL) {
return;
}
BookNode* temp = *head;
if (temp->author == targetAuthor) {
*head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->author != targetAuthor) {
temp = temp->next;
}
if (temp == NULL) {
printf("Element not found.\n");
} else {
BookNode* nextTemp = temp->next;
free(temp);
temp = nextTemp;
}
}
```
5. **求单链表的长度**:
```c
int listLength(BookNode* head) {
int count = 0;
while (head != NULL) {
count++;
head = head->next;
}
return count;
}
```
6. **取表中第i个元素**:
由于链表没有直接的索引访问,你需要从头开始遍历找到第i个元素。这需要修改上面的`printList`函数,增加计数器。
7. **在表中查找值为e的元素位置**:
```c
int findPosition(BookNode* head, int targetAuthor) {
int position = 0;
BookNode* temp = head;
while (temp != NULL) {
if (temp->author == targetAuthor) {
break;
}
temp = temp->next;
position++;
}
return position;
}
```
阅读全文
相关推荐
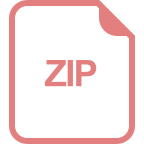
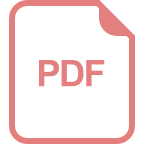
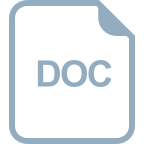
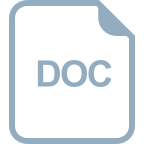
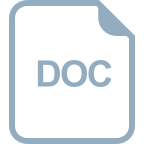
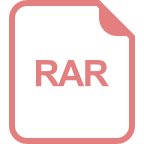
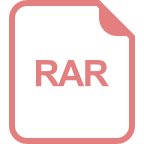
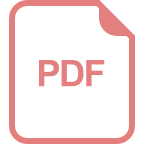
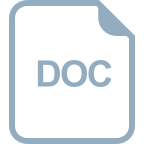
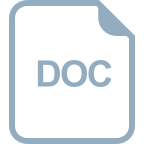
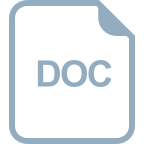
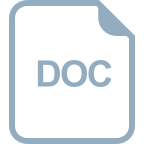
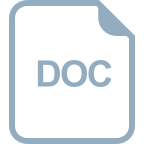
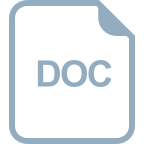
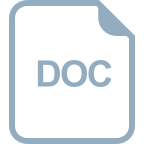
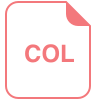