基于多层感知机的趣味实验python代码
时间: 2024-10-16 07:13:27 浏览: 38
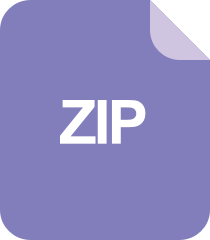
mlp_py_MLP_python_多层感知机_
基于多层感知机(Multilayer Perceptron,MLP)的趣味实验通常涉及神经网络的简单应用,比如图像识别、手写数字识别等。这里我们可以演示一个简单的例子,使用Python和深度学习库Keras实现一个简单的MNIST手写数字识别模型。
```python
# 导入必要的库
import numpy as np
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense
# 加载MNIST数据集
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 数据预处理
train_images = train_images.reshape((60000, 784)) / 255.0
test_images = test_images.reshape((10000, 784)) / 255.0
train_labels = np.eye(10)[train_labels]
test_labels = np.eye(10)[test_labels]
# 创建一个简单的MLP模型
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(64, activation='relu'))
model.add(Dense(10, activation='softmax'))
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=5, validation_split=0.1)
# 测试模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f"Test accuracy: {test_acc * 100}%")
# 趣味部分:随机预测
random_index = np.random.randint(0, len(test_images))
prediction = model.predict_classes(test_images[random_index].reshape(1, -1))
print(f"Predicted digit: {prediction[0]}, Actual digit: {np.argmax(test_labels[random_index])}")
```
这个代码创建了一个三层的神经网络来对MNIST数据集的手写数字进行分类。运行它,你可以看到模型的训练结果,并通过随机预测感受一下多层感知机的基本运作。
阅读全文
相关推荐
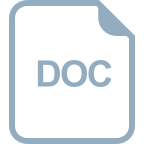
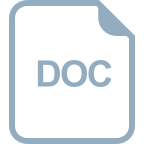
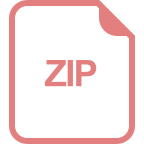
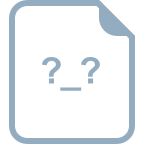
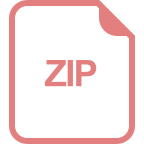
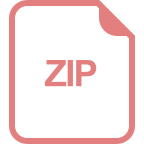
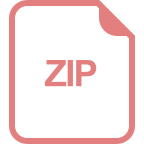
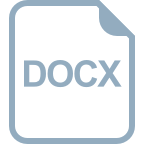
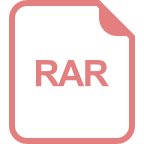
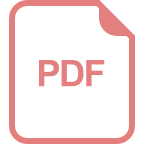
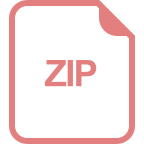
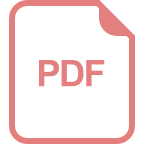