用C语言编写一应用程序,该程序包含三个进程,每个进程中包含两个线程,采用共享内存、套接字、信号量等通信方式实现进程间的通信、同步、互斥操作。
时间: 2024-12-12 18:19:26 浏览: 18
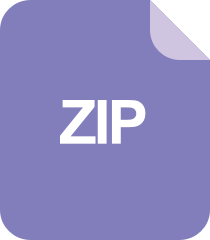
c语言多进程多线程编程
在C语言中,创建一个多进程多线程的应用程序通常会结合`fork()`函数用于创建新进程,`pthread_create()`函数用于创建新线程,并利用各种并发控制机制如共享内存(`shm_open`、`mmap`)、套接字(socket API)以及信号量(`semaphore.h`库)进行通信、同步和互斥。
下面是一个简化的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h> // for shm_key and shmat
#include <sys/shm.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <semaphore.h>
#define SHM_KEY 12345
#define SEMAPHORE_ID 67890
// 创建共享内存区域
int create_shmem(int size) {
key_t shm_key = ftok("shared_mem", 's');
int shm_fd = shmget(shm_key, size, IPC_CREAT | 0666);
return shm_fd;
}
// 创建并初始化信号量
sem_t *create_semaphore() {
sem_init(&sem, 0, 1); // 初始化为1(表示可用)
return &sem;
}
void* thread_func(void* arg) {
int thread_id = (intptr_t)arg; // 线程标识
printf("Thread %d started.\n", thread_id);
// 使用相应通信机制...
return NULL;
}
int main() {
// 创建三个进程
pid_t procs[] = {fork(), fork(), fork()};
if (procs[0] == -1) {
perror("Fork failed");
exit(1);
}
// 对于每个进程,创建两个线程
for (int i = 0; i < 3; i++) {
if (procs[i] > 0) {
pthread_t threads[2];
for (int j = 0; j < 2; j++) {
pthread_create(&threads[j], NULL, thread_func, (void*)(intptr_t)i * 2 + j);
}
// 同步、互斥操作...
sem_wait(create_semaphore()); // 阻塞等待直到其他线程释放信号量
} else {
wait(NULL); // 父进程等待子进程结束
}
}
return 0;
}
```
在这个例子中,你需要自己添加如何在共享内存上读写数据、如何通过套接字建立连接进行通信,以及如何使用信号量进行同步操作的具体代码。
阅读全文
相关推荐
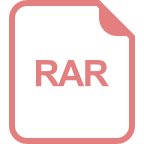
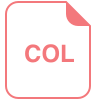
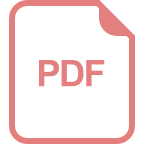
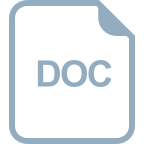
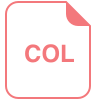
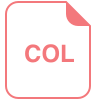
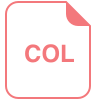
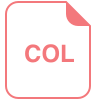
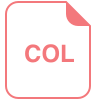
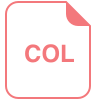
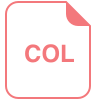
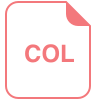
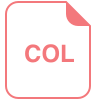
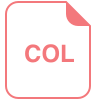
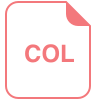
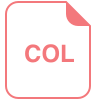
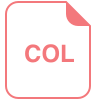