C# bmp转 byte []
时间: 2024-10-12 14:10:36 浏览: 10
在C#中,将Bitmap图像转换为byte数组通常涉及到将图像数据读取到内存,并保存为字节序列。以下是一个简单的步骤示例:
```csharp
using System.Drawing;
using System.IO;
public byte[] BitmapToByteArray(Bitmap bitmap)
{
// 获取Bitmap的数据流
MemoryStream ms = new MemoryStream();
bitmap.Save(ms, ImageFormat.Bmp); // 将Bitmap保存为BMP格式
// 转换为byte数组
ms.Seek(0, SeekOrigin.Begin);
byte[] imageData = new byte[ms.Length];
ms.Read(imageData, 0, (int)ms.Length);
// 关闭流并返回byte数组
ms.Close();
return imageData;
}
```
在这个例子中,我们首先创建一个MemoryStream来存储Bitmap的数据。然后使用`Save`方法将Bitmap转换为BMP格式并写入流中。接着移动流的位置回到开始,以便读取所有数据。最后读取整个流到byte数组中并关闭流。
相关问题
C# bmp转 byte[] 只需要纯像素点数据,比如这张图1920x1080,那这个byte[]大小应该是2073600,请给出正确的代码
在C#中,如果你只需要图片的像素数据(即RGB值),并且不包括BMP文件头和元数据等额外信息,你可以使用System.Drawing.Imaging namespace中的Bitmap类来进行转换。下面是一个示例代码片段,它将读取一张1920x1080的图片并只获取像素数据转换成byte[]:
```csharp
using System.Drawing;
using System.Drawing.Imaging;
public byte[] BitmapToPixelData(Bitmap bitmap)
{
// 确保图像宽度和高度正确
if (bitmap.Width != 1920 || bitmap.Height != 1080)
throw new ArgumentException("Image size is not 1920x1080");
// 创建一个新的位图缓冲区,仅包含像素数据
using (var memoryStream = new MemoryStream())
{
bitmap.Save(memoryStream, ImageFormat.Bgra32); // Bgra32格式包含Alpha通道
memoryStream.Seek(0, SeekOrigin.Begin); // 移动流的位置到开头
var pixelData = new byte[memoryStream.Length];
memoryStream.Read(pixelData, 0, pixelData.Length);
return pixelData;
}
}
// 使用示例
Bitmap myBitmap = new Bitmap("path_to_your_image.bmp");
byte[] pixelArray = BitmapToPixelData(myBitmap);
//
C# bitmap转 byte数组
可以使用 Bitmap 类的 LockBits 方法将 Bitmap 对象转换为 byte 数组。
下面是一个示例代码:
```csharp
Bitmap bmp = new Bitmap("test.bmp");
Rectangle rect = new Rectangle(0, 0, bmp.Width, bmp.Height);
BitmapData bmpData = bmp.LockBits(rect, ImageLockMode.ReadOnly, bmp.PixelFormat);
IntPtr ptr = bmpData.Scan0;
int bytes = Math.Abs(bmpData.Stride) * bmp.Height;
byte[] rgbValues = new byte[bytes];
Marshal.Copy(ptr, rgbValues, 0, bytes);
bmp.UnlockBits(bmpData);
```
在上面的代码中,首先创建一个 Bitmap 对象。然后使用 LockBits 方法获取 BitmapData 对象,该对象包含有关图像位图数据的信息。接下来,我们使用 Marshal.Copy 方法将位图数据从内存中的指针复制到 byte 数组中。最后,使用 UnlockBits 方法释放 BitmapData 对象。
请注意,这只是一个示例代码,具体实现可能需要根据自己的需求进行修改。
阅读全文
相关推荐
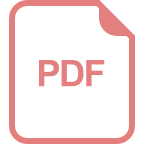
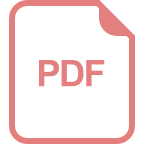
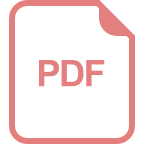













