编码实现凯撒密码的加密和解密操作;加密过程的代码实现
时间: 2024-10-09 15:16:30 浏览: 31
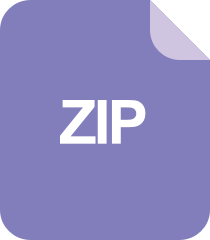
凯撒密码加密和解密python实现源码.zip
凯撒密码的编码实现主要包括加密和解密两个步骤。在C语言中,我们可以创建两个函数:`caesar_encrypt`用于加密,`caesar_decrypt`用于解密,它们的核心都是基于相同的原理:把字母表中的每个字母向右(加密)或向左(解密)移动指定的位置。
下面是一个简单的C语言实现:
```c
#include <stdio.h>
#include <string.h>
// 加密函数
char caesar_encrypt(char c, int shift) {
if ('a' <= c && c <= 'z') {
c = ((c - 'a' + shift) % 26) + 'a';
} else if ('A' <= c && c <= 'Z') {
c = ((c - 'A' + shift) % 26) + 'A';
}
return c;
}
// 解密函数
char caesar_decrypt(char c, int shift) {
return caesar_encrypt(c, -shift); // 将shift变为负数进行解密
}
// 文本加密和显示
void encrypt_text(const char *text, int shift) {
char encrypted[strlen(text)];
for (int i = 0; text[i]; ++i) {
encrypted[i] = caesar_encrypt(text[i], shift);
}
printf("Encrypted Text: %s\n", encrypted);
}
// 文本解密和显示
void decrypt_text(const char *text, int shift) {
char decrypted[strlen(text)];
for (int i = 0; text[i]; ++i) {
decrypted[i] = caesar_decrypt(text[i], shift);
}
printf("Decrypted Text: %s\n", decrypted);
}
int main() {
const char *message = "Hello, World!";
int shift = 3;
encrypt_text(message, shift);
decrypt_text(encrypted_message, shift); // 使用加密后的文本作为解密的输入
return 0;
}
```
在这个代码中,`encrypt_text`函数会接收一段文本和一个密钥值,并生成加密后的结果;`decrypt_text`则是解密操作,只需将密钥取反即可。
阅读全文
相关推荐
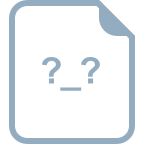
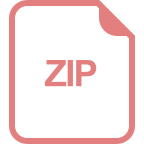

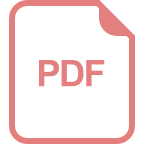
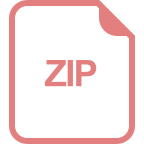
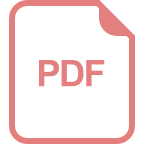
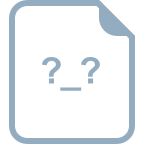
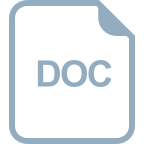
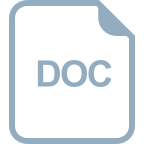
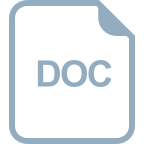
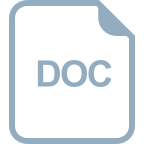
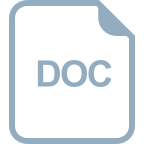
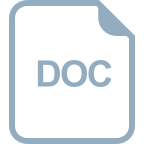
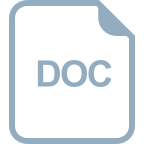
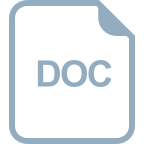
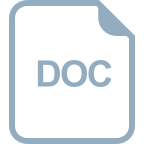

