opencv python 画矩形
时间: 2024-04-15 09:23:16 浏览: 34
在OpenCV中使用Python画矩形可以通过以下步骤实现:
1. 导入OpenCV库:
```python
import cv2
```
2. 读取图像:
```python
image = cv2.imread("image.jpg")
```
这里的"image.jpg"是你要处理的图像文件路径。
3. 定义矩形的位置和大小:
```python
x, y, width, height = 100, 100, 200, 150
```
这里的(x, y)是矩形左上角的坐标,width和height分别是矩形的宽度和高度。
4. 绘制矩形:
```python
cv2.rectangle(image, (x, y), (x + width, y + height), (0, 255, 0), 2)
```
这里的(0, 255, 0)是矩形的颜色,(0, 255, 0)表示绿色。2是矩形边框的宽度。
5. 显示图像:
```python
cv2.imshow("Rectangle", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这里会创建一个名为"Rectangle"的窗口来显示绘制了矩形的图像,并等待用户按下任意键关闭窗口。
以上就是使用OpenCV和Python绘制矩形的基本步骤。
相关问题
python opencv 画矩形
在Python中使用OpenCV库可以很方便地画矩形。你可以使用cv2.rectangle函数来实现。下面是一个示例代码:
```python
import cv2
image = cv2.imread('image.jpg') # 读取图像
x, y, w, h = 100, 100, 200, 150 # 矩形的左上角坐标和宽高
color = (0, 0, 255) # 矩形的颜色,这里使用红色
thickness = 2 # 矩形边框的粗细
cv2.rectangle(image, (x, y), (x + w, y + h), color, thickness) # 画矩形
cv2.imwrite('output.jpg', image) # 保存图像
```
在这个例子中,我们首先使用cv2.imread函数读取了一张图像。然后,我们定义了矩形的左上角坐标和宽高。接下来,我们指定了矩形的颜色和边框的粗细。最后,我们使用cv2.rectangle函数在图像上画出了矩形,并使用cv2.imwrite函数保存了结果图像。
python opencv画矩形
要在图像上绘制矩形,可以使用OpenCV的`cv2.rectangle()`函数。下面是一个简单的示例代码,展示了如何使用OpenCV绘制矩形:
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 定义矩形的起始点和结束点坐标
start_point = (100, 100)
end_point = (300, 300)
# 定义矩形的颜色和线宽
color = (0, 255, 0) # 绿色
thickness = 2
# 在图像上绘制矩形
cv2.rectangle(image, start_point, end_point, color, thickness)
# 显示绘制矩形后的图像
cv2.imshow('Image with Rectangle', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们使用`cv2.rectangle()`函数来绘制一个矩形。`start_point`和`end_point`分别指定矩形的左上角和右下角坐标。`color`指定了矩形的颜色,这里使用了RGB格式,(0, 255, 0)表示绿色。`thickness`指定了绘制矩形的线宽。
最后,通过`cv2.imshow()`函数显示绘制矩形后的图像,并通过`cv2.waitKey(0)`等待用户按下任意键关闭窗口。最后使用`cv2.destroyAllWindows()`关闭所有的窗口。
你可以根据需要调整矩形的起始点、结束点、颜色和线宽来绘制不同样式的矩形。
相关推荐
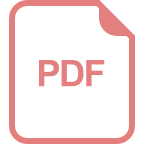
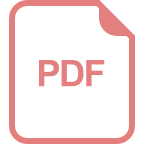












