C++不等长字符串排序
时间: 2024-06-21 19:04:07 浏览: 54
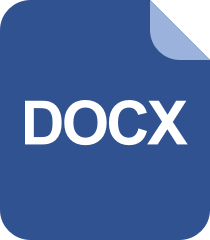
四种方法对字符串排序

在C++中,标准库并没有提供直接支持不等长字符串排序的函数,因为C++的`std::string`类型默认是等长的,即所有的字符数组都有相同的长度。如果你需要对不等长字符串进行排序,通常会用到自定义比较函数或者使用一些第三方库,如`boost`中的`multi_index_container`。
一个常见的做法是定义一个结构体或类,其中包含一个字符串和其长度,然后使用这个复合类型的容器来进行排序。这里是一个简单的例子:
```cpp
#include <string>
#include <vector>
#include <algorithm>
#include <boost/multi_index_container.hpp>
#include <boost/multi_index/ordered_index.hpp>
#include <boost/multi_index/hashed_index.hpp>
// 定义一个结构体,包含字符串和长度
struct StringWithLength {
std::string str;
size_t length;
};
// 自定义比较函数,用于按长度排序
bool compareStringLength(const StringWithLength& a, const StringWithLength& b) {
return a.length < b.length;
}
int main() {
std::vector<StringWithLength> strings = {/*你的字符串列表*/};
// 使用boost的multi_index_container进行排序
boost::multi_index_container<StringWithLength,
boost::multi_index::indexed_by<boost::multi_index::ordered_unique<boost::multi_index::member<StringWithLength, size_t, &StringWithLength::length>>,
boost::multi_index::hashed_unique<boost::multi_index::tag<>, boost::multi_index::member<StringWithLength, std::string const&, &StringWithLength::str>>>>
my_string_list(strings);
std::sort(my_string_list.begin(), my_string_list.end(), compareStringLength);
// 现在我的_string_list已经是按长度升序排列的了
for (const auto& str : my_string_list) {
std::cout << str.str << " (" << str.length << ")\n";
}
return 0;
}
```
阅读全文
相关推荐
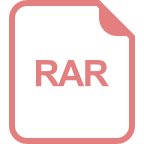
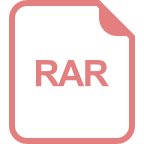










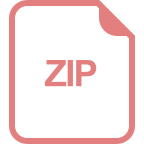
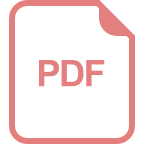
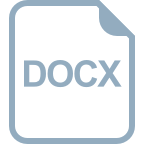
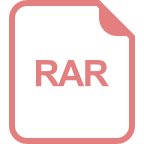
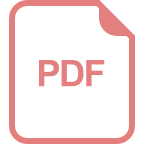
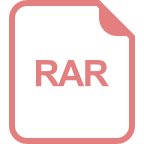