写出完整的C++11代码
时间: 2024-12-22 19:11:36 浏览: 4
为了帮助小明编写C++11代码来解决这个问题,我们可以使用动态规划和优先队列。首先,我们需要定义几个辅助函数和数据结构。以下是完整的C++11代码示例:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
// 定义食物类
struct Food {
int id;
std::vector<int> nutrients; // 每种食物的营养成分
bool contains(std::vector<unsigned short>& required) const {
for (int nutrient : required) {
if (std::find(nutrients.begin(), nutrients.end(), nutrient) == nutrients.end()) return false;
}
return true;
}
};
// 动态规划辅助函数
std::pair<int, std::vector<int>> findBestSolution(const std::vector<Food>& foods, std::vector<unsigned short>& required) {
int maxWeight = std::numeric_limits<int>::max();
std::vector<int> bestFoodIds;
std::priority_queue<std::pair<int, int>, std::vector<std::pair<int, int>>, std::greater<std::pair<int, int>>> pq;
for (int i = 0; i <= 65535; ++i) {
while (!pq.empty() && pq.top().first > i) {
pq.pop();
}
if (!pq.empty() && foods[pq.top().second].contains(required)) {
int weight = foods[pq.top().second].nutrients.size(); // 计算当前食物的重量
maxWeight = std::min(maxWeight, weight);
bestFoodIds.push_back(pq.top().second);
pq.pop();
}
if (i != required.back()) continue; // 跳过不需要的营养
for (size_t foodId = 0; foodId < foods.size(); ++foodId) {
if (foods[foodId].contains({required[i]})) { // 如果食物能满足当前营养
pq.push({foods[foodId].nutrients.size(), foodId});
}
}
}
return {maxWeight >= foods.size() ? -1 : maxWeight, bestFoodIds};
}
int main() {
int n, m;
std::cin >> n >> m;
std::vector<unsigned short> required(n);
for (int i = 0; i < n; ++i) {
std::cin >> required[i];
}
std::vector<Food> foods(m);
for (int i = 0; i < m; ++i) {
int foodNum;
std::cin >> foodNum;
std::vector<int> nutrients;
for (int j = 0; j < n; ++j) {
int nutrient;
std::cin >> nutrient;
if (nutrient != 0) nutrients.push_back(j); // 忽略0,只保留非零营养元素
}
foods[i] = {i, nutrients};
}
auto result = findBestSolution(foods, required);
if (result.first == -1) {
std::cout << "-1" << std::endl;
} else {
for (int foodId : result.second) {
std::cout << foodId << " ";
}
std::cout << std::endl;
}
return 0;
}
```
这个代码首先读取输入的数据,然后定义了`Food`结构体以及动态规划辅助函数`findBestSolution`。函数中使用优先队列存储食物及其营养权重,通过不断调整队列中的食物选择,找到满足营养需求且食物数量最少的方案。`main`函数中调用此辅助函数并输出结果。
阅读全文
相关推荐
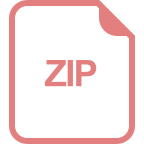
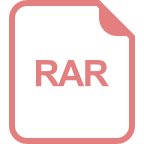
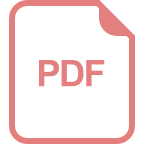
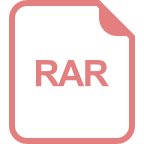
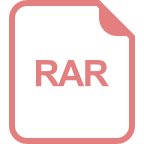
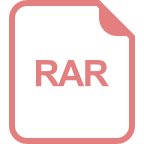
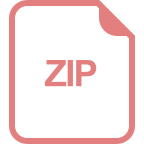
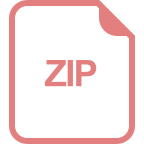
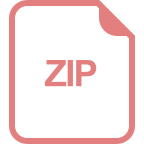
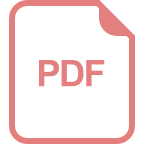
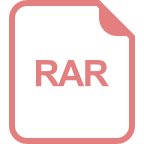
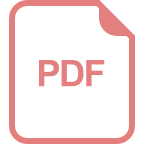
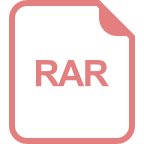
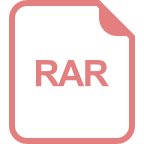
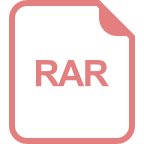
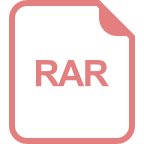
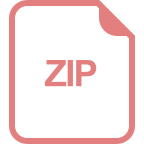
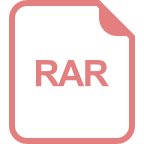