android studio 使用心知天气天气预报app
时间: 2023-12-27 11:25:15 浏览: 31
以下是使用Android Studio和心知天气API创建天气预报应用的步骤:
1. 在Android Studio中创建一个新的项目。
2. 在项目的build.gradle文件中添加以下依赖项:
```groovy
implementation 'com.android.volley:volley:1.2.0'
implementation 'com.google.code.gson:gson:2.8.7'
```
3. 在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
4. 创建一个新的Java类,命名为Weather.java,用于存储天气信息的数据模型。
```java
public class Weather {
private String city;
private String temperature;
private String weather;
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getTemperature() {
return temperature;
}
public void setTemperature(String temperature) {
this.temperature = temperature;
}
public String getWeather() {
return weather;
}
public void setWeather(String weather) {
this.weather = weather;
}
}
```
5. 创建一个新的Java类,命名为WeatherRequest.java,用于发送天气请求并解析JSON响应。
```java
public class WeatherRequest {
private static final String API_KEY = "YOUR_API_KEY";
private static final String BASE_URL = "https://api.seniverse.com/v3/weather/now.json";
public interface WeatherCallback {
void onSuccess(Weather weather);
void onError(String message);
}
public static void getWeather(String city, WeatherCallback callback) {
String url = BASE_URL + "?key=" + API_KEY + "&location=" + city;
JsonObjectRequest request = new JsonObjectRequest(Request.Method.GET, url, null,
response -> {
try {
JSONObject result = response.getJSONObject("results").getJSONArray("now").getJSONObject(0);
Weather weather = new Weather();
weather.setCity(result.getString("location"));
weather.setTemperature(result.getString("temperature"));
weather.setWeather(result.getString("text"));
callback.onSuccess(weather);
} catch (JSONException e) {
callback.onError("Failed to parse JSON response");
}
},
error -> callback.onError("Failed to make weather request"));
RequestQueue queue = Volley.newRequestQueue(context);
queue.add(request);
}
}
```
6. 在MainActivity.java中,添加以下代码来获取天气信息并更新UI:
```java
public class MainActivity extends AppCompatActivity {
private TextView cityTextView;
private TextView temperatureTextView;
private TextView weatherTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
cityTextView = findViewById(R.id.cityTextView);
temperatureTextView = findViewById(R.id.temperatureTextView);
weatherTextView = findViewById(R.id.weatherTextView);
WeatherRequest.getWeather("YOUR_CITY", new WeatherRequest.WeatherCallback() {
@Override
public void onSuccess(Weather weather) {
runOnUiThread(() -> {
cityTextView.setText(weather.getCity());
temperatureTextView.setText(weather.getTemperature());
weatherTextView.setText(weather.getWeather());
});
}
@Override
public void onError(String message) {
runOnUiThread(() -> Toast.makeText(MainActivity.this, message, Toast.LENGTH_SHORT).show());
}
});
}
}
```
请注意,将"YOUR_API_KEY"替换为您在心知天气网站上获取的API密钥,并将"YOUR_CITY"替换为您想要获取天气信息的城市。
相关推荐
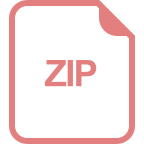
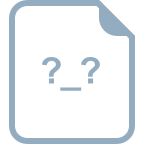














