计算机语言编程绘制2fsk和2psk的调制波形,输入固定十六进制数据0xEB5A,能绘制调制波形
时间: 2024-09-18 08:13:41 浏览: 64
在计算机编程中,特别是使用图形库如Matplotlib(Python)、Processing(Java/PYTHON)或是WPF(Windows Presentation Foundation, .NET)时,可以创建一个程序来绘制二相键控(2FSK)和二进制相移键控(2PSK)的调制波形。这里我将简要概述如何在Python中使用matplotlib库来完成这个任务,假设你已经安装了这个库。
首先,我们需要了解2FSK和2PSK的区别:
- 2FSK:信号通过两个频率的不同交替来表示数字信息,通常使用两个离散的频率,每个频率对应一位数据。
- 2PSK:信号通过改变载波的相位来表示数据,有正相和负相两种状态。
对于输入的固定十六进制数据`0xEB5A`,我们将需要将其转换成二进制序列来进行模拟。
以下是简单的Python代码示例:
```python
import matplotlib.pyplot as plt
import numpy as np
# 固定十六进制数据转换
hex_data = '0xEB5A'
bin_data = ''.join(format(int(hex_char, 16), '04b') for hex_char in hex_data)
# 假设我们有两个不同的频率 f1 和 f2
f1, f2 = 1, 2 # 频率示例
fs = 1000 # 采样率
# 对于2FSK,我们将用不同的频率表示二进制位
def plot_2fsk(binary_data):
t = np.linspace(0, len(bin_data)/fs, len(bin_data))
carrier = np.sin(2 * np.pi * (f1 + f2) * t)
modulated = []
for bit in binary_data:
if bit == '0':
modulated.append(carrier)
else:
modulated.append(-carrier)
plt.plot(t, np.convolve(modulated, [1/2, 1/2]), label='2FSK')
# 对于2PSK,我们将改变载波的相位
def plot_2psk(binary_data):
t = np.linspace(0, len(bin_data)/fs, len(bin_data))
carrier = np.cos(2 * np.pi * f1 * t)
modulated = [carrier*np.exp(1j * 2 * np.pi * phase * (1 if bit == '1' else -1)) for bit, phase in zip(binary_data, range(len(binary_data)))]
plt.plot(t, np.real(modulated), label='2PSK')
# 将十六进制转换为二进制并绘制
binary_data = bin(int.from_bytes(bytes.fromhex(hex_data), 'big'))
plot_2fsk(binary_data)
plot_2psk(binary_data)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.legend()
plt.show()
阅读全文
相关推荐
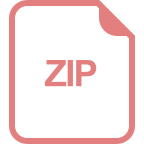
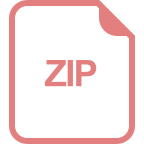
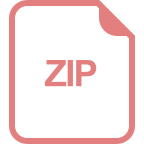



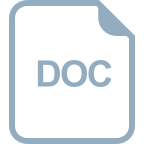
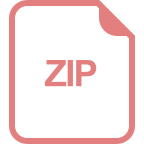
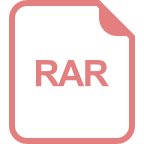
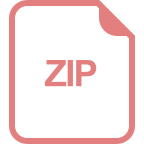
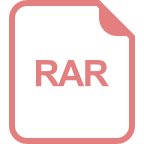
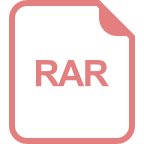
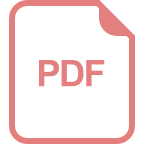
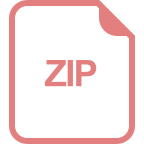
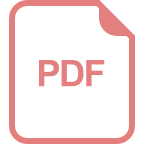
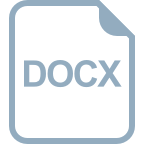
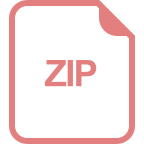
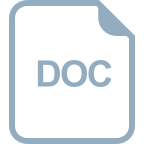