计算某一天是某年的第几天用C++语言表示
时间: 2024-11-13 14:20:04 浏览: 18
在C++中,计算某一天是一年中的第几天可以使用蔡勒公式(Zeller's congruence),这是一种基于日期的算法。下面是一个简单的函数示例:
```cpp
#include <iostream>
#include <vector>
// Zeller's congruence function to find the day of the week
int zellersCongruence(int year, int month, int day) {
// Special cases for February and leap years
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0))) {
// February in a leap year has 29 days
return (day + ((13 * (month + 1)) / 5) + year + ((year / 4) - (year / 100) + (year / 400)) % 7);
} else {
// Normal months have 30 or 31 days
return (day + ((13 * (month + 1)) / 5) + year + ((year / 4) - (year / 100)) % 7);
}
}
// Function to find the day number (1-based) from January 1 of that year
int dayOfYear(int year, int month, int day) {
int baseDay = zellersCongruence(year, 1, 1); // Day of the first day of the year
return (zellersCongruence(year, month, day) - baseDay + 1);
}
int main() {
int year, month, day;
std::cout << "Enter the year, month (1-12), and day: ";
std::cin >> year >> month >> day;
int dayNumber = dayOfYear(year, month, day);
std::cout << "The day is " << dayNumber << " out of 365 (or 366 in a leap year)\n";
return 0;
}
```
当你运行这个程序并输入具体的年月日,它会返回该天是一年中的第几天。注意这只是一个基本实现,实际应用中可能需要考虑闰年的情况。
阅读全文
相关推荐
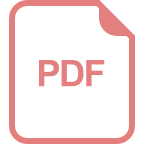
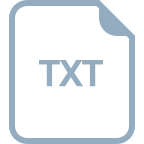
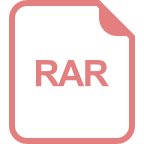















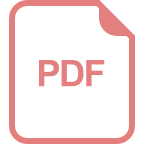
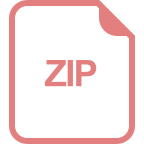