c语言从文件中顺序读取四字节,并对每个四字节进行不同条件的判断
时间: 2024-09-26 11:16:56 浏览: 44
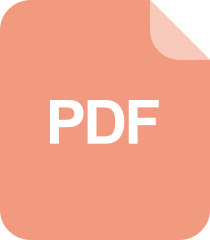
用C语言实现从文本文件中读取数据后进行排序的功能
在C语言中,你可以通过`fread`函数从文件中顺序读取四个字节的数据,然后使用`switch-case`语句或者一系列的条件判断来进行处理。下面是一个简单的示例:
```c
#include <stdio.h>
int main() {
FILE *file = fopen("your_file.txt", "rb"); // 打开二进制模式读取文件
if (file == NULL) {
perror("Failed to open file");
return 1;
}
unsigned char bytes[4]; // 四字节数据数组
// 一次读取四个字节
size_t num_read = fread(bytes, sizeof(unsigned char), 4, file);
if (num_read != 4) {
printf("Error reading from file\n");
fclose(file);
return 1;
}
fclose(file); // 关闭文件后不再需要
// 对每个字节进行判断
switch (bytes[0]) {
case 'A':
// 对第一个字节值为'A'的情况处理
break;
case 'B':
// 对第二个字节值为'B'的情况处理
break;
case 'C':
// 对第三个字节值为'C'的情况处理
break;
default:
// 其他情况的处理
printf("Unknown value: %d\n", bytes[0]);
}
// 类似地,可以添加更多case分支来处理其他可能的字节组合
return 0;
}
```
在这个例子中,我们首先打开文件并检查是否成功,然后读取四个字节到`bytes`数组中。接着,使用`switch`结构按第一个字节的不同值进行相应的操作。记得在处理完毕后关闭文件。
阅读全文
相关推荐
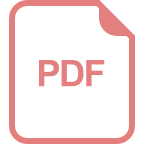
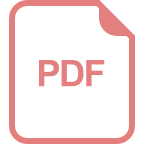
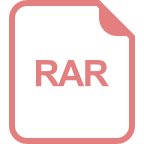
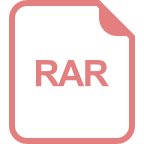
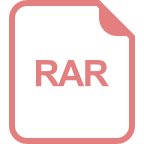
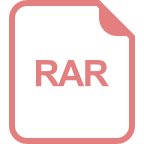
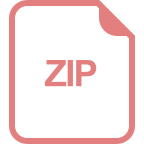
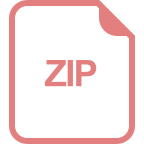
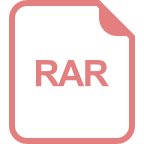
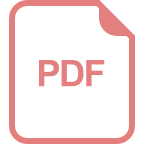
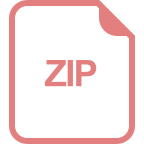