给出完整的c语言代码解决问题
时间: 2024-12-20 17:12:10 浏览: 6
当然可以,这里是一个完整的C语言代码示例,它实现了上述描述的短作业优先算法,并按照到达时间对作业进行调度:
```c
#include <stdio.h>
#include <stdlib.h>
#include <queue>
// 定义作业结构体
typedef struct {
int id;
int runtime;
int arrival_time;
} Job;
// 比较函数,用于优先队列排序
int compareJobs(const void* a, const void* b) {
const Job *jobA = (const Job *)a;
const Job *jobB = (const Job *)b;
return jobB->arrival_time - jobA->arrival_time; // 按照到达时间升序排列
}
int main() {
// 作业数据
Job jobs[] = {
{1, 700, 1},
{2, 800, 50},
{3, 815, 30},
{4, 820, 5},
{5, 830, 25},
{6, 835, 20},
{7, 845, 15}
};
int numJobs = sizeof(jobs) / sizeof(jobs[0]);
// 创建优先队列
std::priority_queue<Job*, std::vector<Job*>, decltype(&compareJobs)> pq(&compareJobs);
// 添加作业到队列
for (int i = 0; i < numJobs; i++) {
pq.push(&jobs[i]);
}
// 调度并输出结果
long currentTime = 0;
while (!pq.empty()) {
Job *currentJob = pq.top();
pq.pop();
currentTime = currentJob->arrival_time;
printf("Processing Job %d with runtime of %d seconds...\n", currentJob->id, currentJob->runtime);
currentTime += currentJob->runtime;
printf("Job %d finished at time %ld\n", currentJob->id, currentTime);
}
return 0;
}
```
这个代码首先创建了一个作业结构体,然后使用`std::priority_queue`作为优先级队列。在主函数中,按照到达时间添加作业,然后不断从队列中取出并执行最小优先级的作业,直到队列为空。
阅读全文
相关推荐
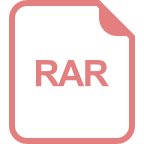
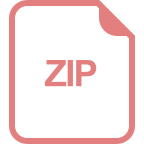
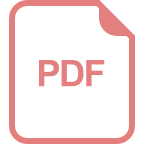
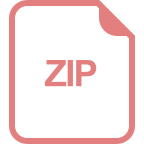
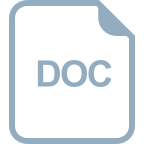
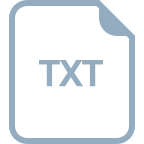
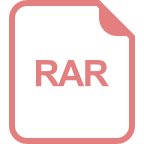
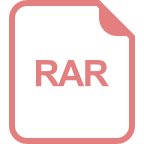
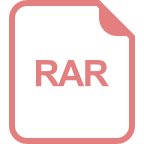
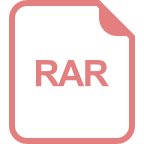
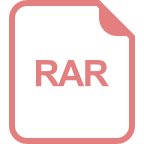
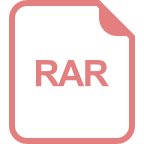
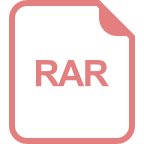
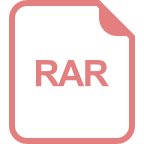
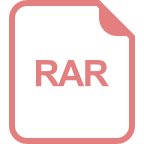
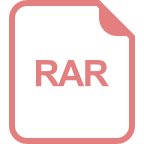