如何使用C语言编程实现日期顺序的转换,即将给定的年-日格式转换为月-日-年格式?
时间: 2024-11-22 09:48:09 浏览: 4
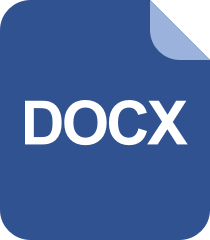
C语言-顺序栈实现十进制转换为二进制-八进制-十六进制
在C语言中,你可以通过以下几个步骤来实现这种日期格式的转换:
1. 定义两个结构体,一个用于存储原格式的日期(year-day),另一个用于存储目标格式的日期(month-day-year)。
```c
struct Date {
int year;
int day;
};
struct ConvertedDate {
int month;
int day;
int year;
};
```
2. 创建函数来接收输入的日期,并根据需要进行计算。例如,你可以假设默认月份的天数,然后调整到正确的月份。这里我们使用内建的`#include <stdio.h>`中的`january`和`days_in_month`作为示例:
```c
int days_in_month(int month, int year) {
if (month == 2) {
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
return 29; // 虚拟闰年
} else {
return 28;
}
} else {
switch(month) {
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
}
void convert_date_format(struct Date input, struct ConvertedDate* output) {
int days_left = input.day - 1; // 减一是因为索引从0开始
int current_month = 0;
while (days_left > 0) {
if (days_left >= days_in_month(current_month + 1, input.year)) {
output->day = days_left;
output->month = current_month + 1;
output->year = input.year;
return;
} else {
days_left -= days_in_month(current_month, input.year);
current_month++;
}
}
}
```
3. 调用这个函数并打印结果:
```c
int main() {
struct Date input = {2023, 366}; // 输入的年-日格式
struct ConvertedDate converted;
convert_date_format(input, &converted);
printf("Converted date: %d-%d-%d\n", converted.month, converted.day, converted.year);
return 0;
}
```
请注意,上述代码仅适用于公历,且没有处理特殊情况如非闰年的2月29日。在实际应用中,你可能需要更全面的日期处理库来处理这些问题。
阅读全文
相关推荐
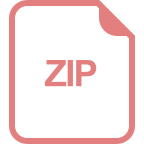
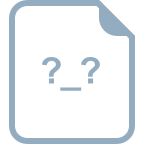
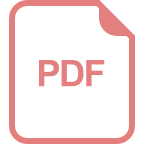
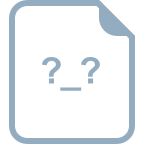
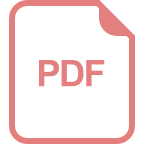
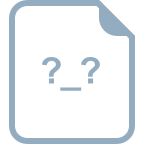
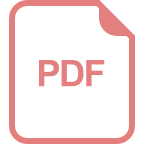
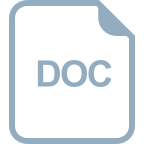
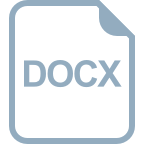
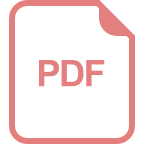
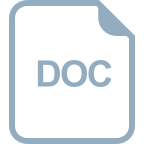
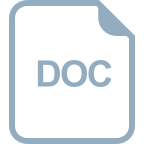
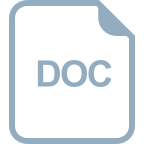
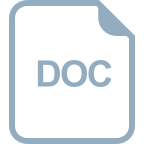
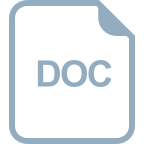
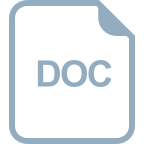
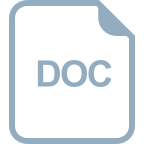
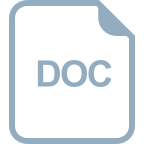