对于环形链表,用c++编写函数求出其中环的长度
时间: 2024-10-12 08:07:41 浏览: 27
环形链表是一种特殊的链表结构,在这种链表中,某个节点的next指针指向了链表中的另一个节点形成了一个环。要在C++中计算环的长度,我们可以使用“快慢指针”算法,这是一种常见的解决环形链表问题的方法。
下面是一个简单的示例,定义了一个`ListNode`结构体表示链表节点,并提供了一个名为`detectCycleLength`的函数来计算环的长度:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
// 检测链表是否有环,若有返回true并初始化fast和slow指针
bool hasCycle(ListNode* head) {
if (head == nullptr || head->next == nullptr) return false;
ListNode slow(*head);
ListNode fast(*head);
while (fast.next != nullptr && fast.next->next != nullptr) {
slow = slow.next;
fast = fast.next->next;
if (slow == fast) { // 如果找到环,fast回到环起点
fast = head;
while (slow != fast) {
slow = slow.next;
fast = fast.next;
}
break;
}
}
return slow == fast; // 如果slow等于fast,则有环;反之则无环
}
// 计算环的长度
int detectCycleLength(ListNode* head) {
if (!hasCycle(head)) return -1; // 若无环,返回-1
int length = 0;
ListNode *slow = head;
ListNode *fast = head;
while (fast != slow) {
slow = slow.next;
fast = fast.next->next;
length++;
}
return length; // 返回环的长度
}
int main() {
// 创建一个环形链表示例,这里省略实际构造过程
ListNode *ringHead = ...;
if (detectCycleLength(ringHead) != -1) {
cout << "环的长度是: " << detectCycleLength(ringHead) << endl;
} else {
cout << "链表无环" << endl;
}
return 0;
}
```
阅读全文
相关推荐
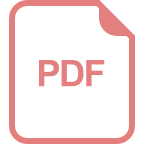
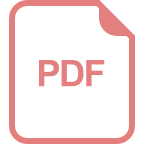
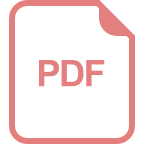
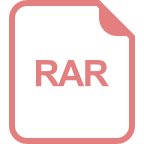
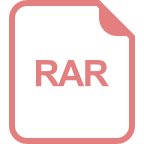
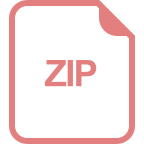
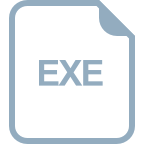
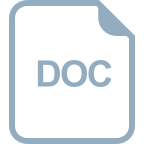
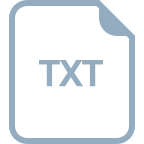
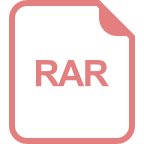
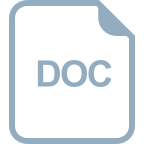
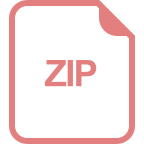
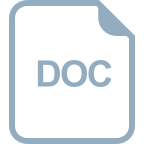