Python海龟代码
时间: 2024-06-10 20:03:32 浏览: 31
Python Turtle模块是一个非常有趣的图形库,它允许你在Python中创建简单的命令式绘图,通常用于教学编程和可视化概念。Turtle就像是一个在屏幕上的小乌龟,你可以给它发送一系列指令,让它在屏幕上画出各种形状。
基本使用海龟代码的例子如下:
```python
import turtle
# 创建一个Turtle对象
t = turtle.Turtle()
# 绘制一个正方形
t.forward(100) # 向前移动100像素
t.right(90) # 右转90度
t.forward(100)
t.right(90)
t.forward(100)
t.right(90)
t.forward(100)
# 结束绘制并关闭窗口
turtle.done()
```
在这个例子中,`forward()`让乌龟向前移动,`right()`则是让乌龟旋转。`turtle.done()`是程序等待用户关闭窗口或按下某个键后才会停止。
相关问题
python的海龟代码
Python 的 Turtle 模块是一个图形绘制工具,它提供了一个简单的命令行接口,让你可以用类似 Logo 语言的方式来创建图形。Turtle 模块中的海龟对象可以移动、旋转、绘制线条和图形,非常适合初学者学习编程中的基本图形和动画概念。
以下是一个简单的 Python 海龟代码示例,用来画一个五边形:
```python
import turtle
# 创建一个海龟对象
t = turtle.Turtle()
t.speed(0) # 设置海龟移动速度(0表示最快)
# 绘制五边形
for _ in range(5):
t.forward(100) # 前进100像素
t.right(72) # 旋转72度(一个完整的圆是360度,五边形每个内角是72度)
# 结束绘制并隐藏海龟
t.hideturtle()
turtle.done() # 显示turtle窗口,直到关闭
python海龟编程代码大全
### 回答1:
下面是一些Python海龟绘图的例子,涵盖了各种形状和图案,供您参考。
1. 绘制一个正方形:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制正方形
for i in range(4):
t.forward(100)
t.right(90)
# 等待退出
turtle.done()
```
2. 绘制一个圆形:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制圆形
t.circle(50)
# 等待退出
turtle.done()
```
3. 绘制一个五角星:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制五角星
for i in range(5):
t.forward(100)
t.right(144)
# 等待退出
turtle.done()
```
4. 绘制一个螺旋线:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制螺旋线
for i in range(100):
t.forward(i)
t.right(20)
# 等待退出
turtle.done()
```
5. 绘制一个彩色螺旋线:
```python
import turtle
import random
# 创建海龟对象
t = turtle.Turtle()
# 绘制彩色螺旋线
for i in range(100):
# 随机生成RGB颜色值
r = random.random()
g = random.random()
b = random.random()
t.color(r, g, b)
t.forward(i)
t.right(20)
# 等待退出
turtle.done()
```
6. 绘制一个心形:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制心形
t.left(45)
t.forward(100)
t.right(90)
t.forward(100)
t.right(180)
t.forward(50)
t.left(90)
t.forward(50)
t.right(180)
t.forward(100)
t.right(180)
# 等待退出
turtle.done()
```
7. 绘制一个棋盘:
```python
import turtle
# 创建海龟对象
t = turtle.Turtle()
# 绘制棋盘
for i in range(8):
for j in range(8):
if (i + j) % 2 == 0:
t.begin_fill()
t.fillcolor("black")
else:
t.begin_fill()
t.fillcolor("white")
t.penup()
t.goto(i * 50, j * 50)
t.pendown()
t.goto(i * 50 + 50, j * 50)
t.goto(i * 50 + 50, j * 50 + 50)
t.goto(i * 50, j * 50 + 50)
t.goto(i * 50, j * 50)
t.end_fill()
# 等待退出
turtle.done()
```
以上就是一些Python海龟编程的例子,希望对您有所帮助。
### 回答2:
Python海龟编程是一种基于图形的编程语言,它通过控制虚拟海龟来绘制图形。下面是一些常见的Python海龟编程代码示例:
1. 在屏幕上绘制一个正方形:
```python
import turtle
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.done()
```
2. 在屏幕上绘制一个圆形:
```python
import turtle
turtle.circle(100)
turtle.done()
```
3. 在屏幕上绘制一个多边形:
```python
import turtle
sides = 6 # 修改这个值可以绘制任意边数的多边形
angle = 360 / sides
for _ in range(sides):
turtle.forward(100)
turtle.right(angle)
turtle.done()
```
4. 在屏幕上绘制一条彩色螺旋线:
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(200):
turtle.pencolor(colors[i % 6])
turtle.width(i/100 + 1)
turtle.forward(i)
turtle.left(59)
turtle.done()
```
这些示例只是Python海龟编程中的一部分代码,还有许多其他有趣的图形可以绘制。希望这些示例能为你提供一些启发,让你能够更好地掌握Python海龟编程。
### 回答3:
《Python海龟编程代码大全》是一本以海龟图形进行编程指导的书籍。这本书包含了丰富的Python代码示例和详细的讲解,能够帮助读者掌握Python海龟图形编程的基本原理和技巧。
书中首先介绍了Python海龟图形编程的基础知识,包括如何创建海龟窗口、设置画布大小和背景颜色等。接下来,书中逐步介绍了海龟图形编程的各种功能和操作,如画直线、画圆、填充颜色等。每个功能都有相应的代码示例和详细的讲解,读者可以通过跟随示例代码进行实践,加深对编程概念和语法的理解。
此外,书中还介绍了一些高级的海龟图形编程技巧,包括如何使用循环和条件语句进行复杂的图形绘制,如何创建自定义的图形函数和类等。这些技巧能够帮助读者更加灵活地控制海龟的移动和图形的绘制,实现更加有趣和复杂的图形效果。
除了基础知识和技巧的讲解,书中还提供了一些实用的项目示例,如绘制太阳花、绘制螺旋图等。这些项目旨在通过实例演示将所学的知识应用到实际问题中的过程,帮助读者培养编程解决问题的能力和思维模式。
总体来说,《Python海龟编程代码大全》是一本全面而详实的Python海龟图形编程入门指南。通过学习这本书,读者可以系统地掌握Python海龟编程的基本原理和技巧,并能够自己编写简单到复杂的海龟图形代码。无论是初学者还是有一定编程经验的读者,都能从这本书中受益匪浅。
相关推荐
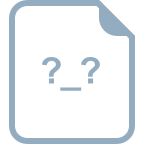












