dijkstra路径规划matlab栅格
时间: 2023-10-09 16:17:18 浏览: 211
Dijkstra算法是一种用于在图中找到最短路径的经典算法。在Matlab中进行栅格路径规划,你可以按照以下步骤实现Dijkstra算法:
1. 创建一个栅格地图,并为每个栅格分配代表其状态的值(例如,障碍物为1,可通过的路径为0)。
2. 初始化起点的距离为0,将其添加到一个待处理节点的集合中。
3. 初始化所有其他节点的距离为无穷大,并将它们添加到一个未访问节点的集合中。
4. 当存在未访问节点时,选择距离起点最近的节点,并将其标记为已访问。
5. 对于当前节点的所有邻居节点,计算通过当前节点到达邻居节点的距离,并更新邻居节点的距离。
6. 重复步骤4和5,直到到达终点或未访问节点集合为空。
7. 从终点开始,按照每个节点的最小距离反向遍历,即可得到最短路径。
以下是一个简单的实现示例:
```matlab
function shortestPath = dijkstra(grid, start, goal)
[n, m] = size(grid);
unvisited = true(n, m);
distance = Inf(n, m);
prev = zeros(n, m);
distance(start) = 0;
while any(unvisited(:))
[~, idx] = min(distance(unvisited));
[i, j] = ind2sub([n, m], idx);
if [i, j] == goal
break;
end
unvisited(i, j) = false;
neighbors = getNeighbors(i, j, n, m);
for k = 1:length(neighbors)
neighbor = neighbors(k);
if grid(neighbor) == 0
alt = distance(i, j) + 1; % Assume each grid has a cost of 1
if alt < distance(neighbor)
distance(neighbor) = alt;
prev(neighbor) = idx;
end
end
end
end
% Reconstruct shortest path
shortestPath = [];
current = sub2ind([n, m], goal(1), goal(2));
while current ~= 0
shortestPath = [shortestPath; current];
current = prev(current);
end
shortestPath = flip(shortestPath);
end
function neighbors = getNeighbors(i, j, n, m)
directions = [-1, 0; 1, 0; 0, -1; 0, 1]; % Up, down, left, right
neighbors = [];
for k = 1:size(directions, 1)
ni = i + directions(k, 1);
nj = j + directions(k, 2);
if ni >= 1 && ni <= n && nj >= 1 && nj <= m
neighbors = [neighbors; sub2ind([n, m], ni, nj)];
end
end
end
```
可以根据你的具体需求对此示例进行修改和扩展。
阅读全文
相关推荐
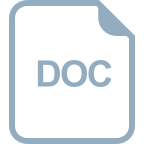
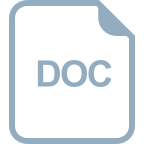
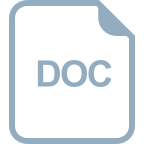
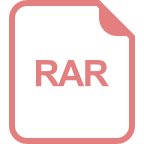



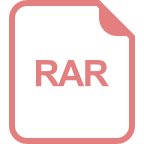
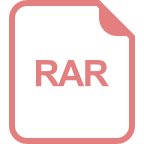
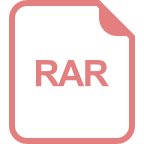
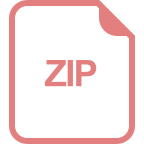
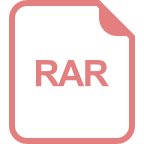
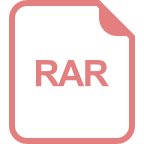
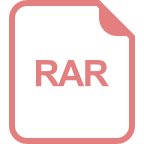
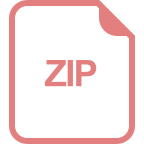
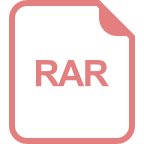
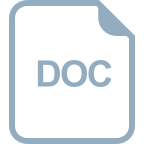

