C++中不使用库函数,编写程序实现字符串的字串匹配
时间: 2024-12-22 13:14:53 浏览: 5
在C++中,不使用库函数直接实现字符串的字串匹配,通常可以使用滑动窗口算法或者朴素的逐字符比较法。这里我们以逐字符比较为例:
1. **逐字符比较**(Brute Force Approach):
- 定义两个指针,一个指向主字符串的开始(i),另一个指向模式串的开始(j = 0)。
- 循环遍历主字符串,对于每个位置i:
- 如果主字符串中的字符与模式串的字符相同(str[i] == pattern[j]),则移动模式串指针j向前一位。
- 如果模式串结束(j == pattern.length()),说明找到匹配,记录下起始位置i。
- 如果主字符串字符与模式串当前字符不同,且模式串指针不是第一个字符,那么回退模式串指针j到上次匹配的位置,继续比较。
- 这种方法时间复杂度较高,是O(n*m),其中n是主字符串长度,m是模式串长度。
```cpp
#include <iostream>
using namespace std;
bool matchString(const string& str, const string& pattern) {
int i = 0, j = 0;
while (i < str.length()) {
if (str[i] == pattern[j]) {
++i;
++j;
} else if (j > 0) {
j = 0;
} else {
++i;
}
}
return j == pattern.length();
}
int main() {
string str = "hello world";
string pattern = "world";
if (matchString(str, pattern)) {
cout << "Pattern found!" << endl;
} else {
cout << "Pattern not found." << endl;
}
return 0;
}
```
阅读全文
相关推荐
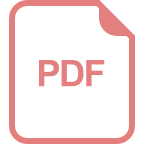
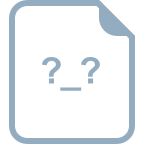
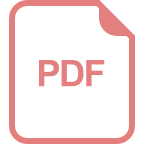
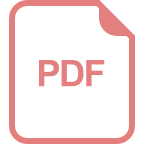
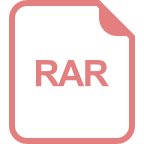
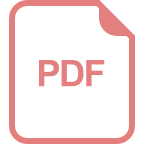
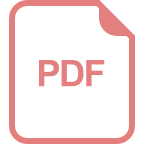
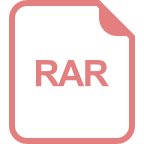
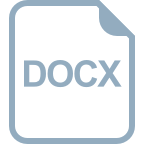
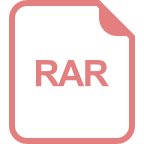
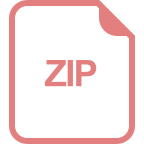
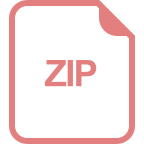
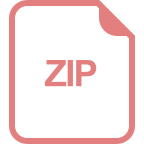