c语言定义一个整型数组array和一个整数target,找出数组array中两个数的和与乘为target的所有组合。
时间: 2024-10-06 10:03:54 浏览: 34
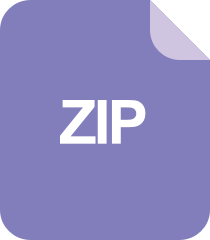
c语言入门编程之数组操作两数之和.zip
在C语言中,你可以使用两层循环以及一个哈希集合(如`unordered_set`)来解决这个问题。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <unordered_set>
// 定义结构体来存储元素和其索引
typedef struct {
int num;
int index;
} Element;
// 函数用于查找数组中两个数的和等于目标值的组合
void find_combinations(int array[], int n, int target) {
std::unordered_set<int> sum_hash;
for (int i = 0; i < n; i++) {
int complement = target - array[i];
// 如果互补的数已经存在,找到了一对和为目标的数
if (sum_hash.find(complement) != sum_hash.end()) {
printf("Pair found: (%d, %d) with sum %d\n",
array[i], complement, target);
}
// 将当前元素添加到哈希集中
sum_hash.insert(array[i]);
}
}
int main() {
int array[] = {2, 7, 11, 15};
int n = sizeof(array) / sizeof(array[0]);
int target = 9;
find_combinations(array, n, target);
return 0;
}
```
在这个程序中,我们遍历整个数组,对于每个元素,我们在`sum_hash`中查找是否存在目标值减去该元素的结果。如果找到,我们就找到了一组和为目标的数;同时将当前元素添加到哈希集合中以便后续检查其他元素。
阅读全文
相关推荐
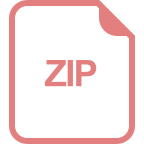
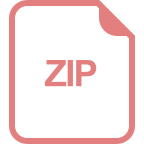















