那么如何写一个静态服务器呢?
时间: 2024-04-13 22:29:18 浏览: 78
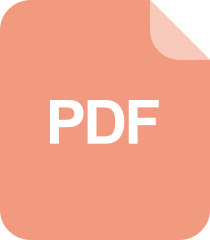
python实现静态web服务器
为了实现一个简单的静态服务器,你可以使用以下步骤:
1. 导入所需的依赖库(如`http`和`fs`)。
2. 创建一个 HTTP 服务器对象。
3. 监听指定的端口号,以便服务器能够接收请求。
4. 在请求到达时,解析请求中的 URL,并获取请求的文件路径。
5. 使用 `fs` 模块读取文件内容。
6. 根据文件类型设置正确的响应头。
7. 将文件内容作为响应主体发送回客户端。
8. 处理错误情况,如文件不存在或读取文件时出错。
这是一个简单的示例代码,展示了如何创建一个静态服务器:
```javascript
const http = require('http');
const fs = require('fs');
const path = require('path');
const server = http.createServer((req, res) => {
// 解析请求的 URL 并获取文件路径
const filePath = path.join(__dirname, req.url);
// 使用 fs 模块读取文件内容
fs.readFile(filePath, (err, data) => {
if (err) {
// 处理文件不存在或读取错误的情况
res.writeHead(404);
res.end('File not found!');
} else {
// 根据文件类型设置正确的响应头
const extname = path.extname(filePath);
let contentType = 'text/plain';
if (extname === '.html') {
contentType = 'text/html';
} else if (extname === '.css') {
contentType = 'text/css';
} else if (extname === '.js') {
contentType = 'text/javascript';
}
// 发送响应
res.writeHead(200, { 'Content-Type': contentType });
res.end(data);
}
});
});
const port = 3000;
server.listen(port, () => {
console.log(`Server running on port ${port}`);
});
```
你可以将上述代码保存为 `.js` 文件并运行,然后在浏览器中访问 `http://localhost:3000`,服务器将返回相应的静态文件。请注意,这只是一个简单的示例,不适用于生产环境,生产环境中通常会有更多的安全和性能考虑。
阅读全文
相关推荐
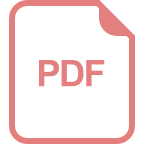
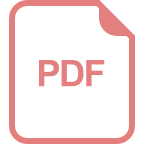
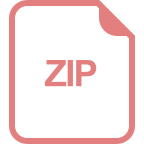
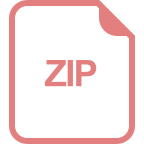
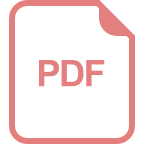
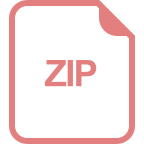
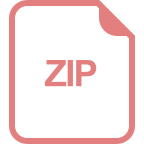
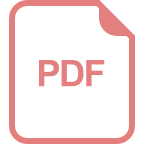
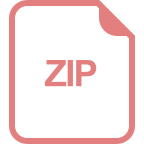
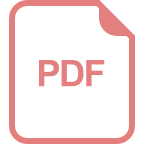
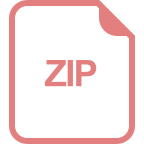
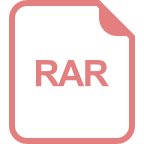
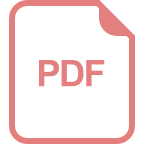
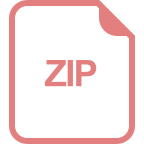
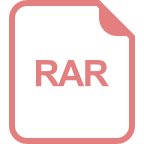
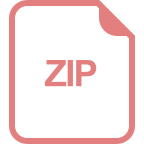