安卓实现button 点击Notification通知栏自动弹出消息 点击消息弹出取消或确认实现Java实例代码
时间: 2024-10-23 12:09:46 浏览: 26
在Android中,为了实现当用户点击Notification通知栏时,能够自动弹出一个对话框并提供取消或确认选项,你需要创建一个Service来发送Notification,并在点击监听器中处理用户的操作。以下是一个简单的Java示例,假设你正在使用`NotificationCompat.Builder`:
```java
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import androidx.core.app.NotificationCompat;
public class MyNotificationService extends Service {
@Override
public void onCreate() {
super.onCreate();
// 创建通知渠道,如果不存在则新建
createNotificationChannels();
// 创建Notification
Intent intent = new Intent(this, MainActivity.class); // 主活动类
intent.addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP | Intent.FLAG_ACTIVITY_NEW_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, 0);
Notification notification = new NotificationCompat.Builder(this, "channel_id")
.setContentTitle("标题")
.setContentText("内容")
.setSmallIcon(R.drawable.ic_notification) // 图标
.setContentIntent(pendingIntent)
.build();
// 当点击通知时触发的意图
pendingIntent.setContentIntent(PendingIntent.getBroadcast(
this,
0,
new Intent(this, NotificationClickReceiver.class),
PendingIntent.FLAG_UPDATE_CURRENT));
// 发送Notification
startForeground(1, notification); // 使用startForeground方法高亮显示
}
private void createNotificationChannels() {
String channelId = "channel_id";
String channelName = "我的频道";
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(channelId, channelName, NotificationManager.IMPORTANCE_DEFAULT);
channel.setDescription("这是描述");
((NotificationManager) getSystemService(NOTIFICATION_SERVICE)).createNotificationChannel(channel);
}
}
// 对于Notification点击事件的接收者
public static class NotificationClickReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
if (intent.getAction().equals(Notification.ACTION_NOTIFICATION_CLICKED)) {
int id = intent.getIntExtra(Notification.EXTRA_NOTIFICATION_ID, -1);
showConfirmationDialog(id); // 显示确认/取消对话框
}
}
private void showConfirmationDialog(int notificationId) {
// 创建一个对话框,可以使用AlertDialog或自定义DialogFragment
AlertDialog alertDialog = new AlertDialog.Builder(context)
.setTitle("提示")
.setMessage("您确定要做这个操作吗?")
.setPositiveButton("确认", (dialog, which) -> {
// 用户选择确认,这里做相应操作
})
.setNegativeButton("取消", null)
.create();
alertDialog.show();
}
}
}
```
在这个例子中,`MyNotificationService`服务负责发送通知,而`NotificationClickReceiver`会在用户点击通知时接收并展示对话框。用户可以选择取消或确认。
阅读全文
相关推荐
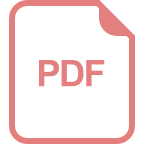
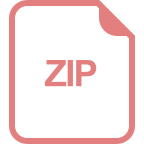
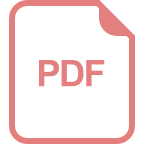
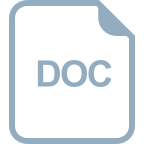
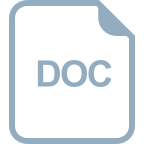
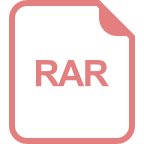
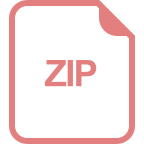
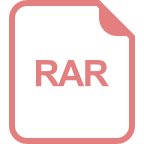
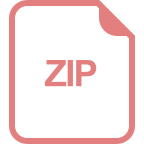
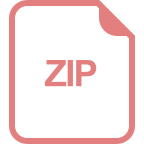
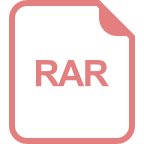
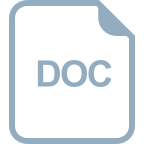
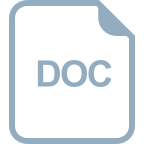
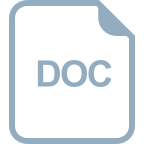
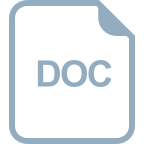
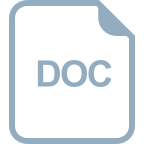
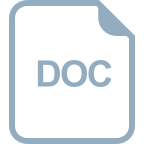
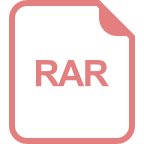