flutter自定义组件 树状图
时间: 2023-07-30 21:11:31 浏览: 86
在 Flutter 中,你可以使用自定义组件来创建树状图。下面是一个简单的示例:
首先,创建一个名为 `TreeNode` 的自定义组件,用于表示树的节点:
```dart
class TreeNode extends StatelessWidget {
final String title;
final List<TreeNode> children;
TreeNode({required this.title, required this.children});
@override
Widget build(BuildContext context) {
return Column(
children: [
Text(title),
SizedBox(height: 10),
Column(
children: children.map((node) => TreeNode(title: node.title, children: node.children)).toList(),
),
],
);
}
}
```
然后,可以使用 `TreeNode` 组件来构建树状图。例如,假设我们有以下的树结构:
```
- Root
- Node 1
- Leaf 1.1
- Leaf 1.2
- Node 2
- Leaf 2.1
- Node 3
```
可以使用以下代码来构建树状图:
```dart
TreeNode root = TreeNode(
title: 'Root',
children: [
TreeNode(
title: 'Node 1',
children: [
TreeNode(title: 'Leaf 1.1', children: []),
TreeNode(title: 'Leaf 1.2', children: []),
],
),
TreeNode(
title: 'Node 2',
children: [
TreeNode(title: 'Leaf 2.1', children: []),
],
),
TreeNode(title: 'Node 3', children: []),
],
);
```
最后,将 `root` 组件放入 `build` 方法中的 `Widget` 树中进行渲染:
```dart
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Tree Example'),
),
body: SingleChildScrollView(
child: TreeNode(title: root.title, children: root.children),
),
);
}
```
这样,你就可以在 Flutter 应用中显示一个简单的树状图了。根据实际需求,你可以对 `TreeNode` 组件进行更多的自定义和样式调整。
相关推荐
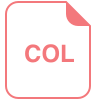
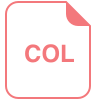
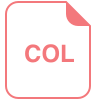
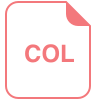
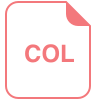









