用c++的string写代码打印以下图案: * * * * * * * * * * * * * * * * * * * * * * * * *
时间: 2024-05-12 22:12:20 浏览: 22
以下是使用C++的string输出图案的代码,题目要求中的空格是由字符串的构造函数和append方法产生的:
```cpp
#include <iostream>
#include <string>
int main()
{
std::string space = " ";
std::string star = "* ";
for (int i = 0; i < 5; i++)
{
std::string output = space;
output.append(i * 2, space);
output.append(5 - i, star);
std::cout << output << std::endl;
}
return 0;
}
```
输出结果:
```
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
```
相关问题
用c++用string打印以下图案: * * * * * * * * * * * * * * * * * * * * * * * * *
以下是用C++中的string打印该图案的代码:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
int n = 5; // 控制行数
int m = 0; // 控制空格数
for (int i = 0; i < n; i++) {
string line = ""; // 每行输出的字符串
for (int j = 0; j < m; j++) {
line += " ";
}
for (int k = 0; k < n; k++) {
line += "* ";
}
cout << line << endl;
m++; // 空格数加1
}
return 0;
}
```
c++代码打印以图案: * * * * * * * * * * * * * * * * * * * * * * * * * string方法。
以下是C++代码打印星号图案的范例:
引用:【C++】用C++实现输出*型图案。代码如下(示例):
```c++
#include <iostream>
using namespace std;
int main() {
int i, k;
for (i = 1; i <= 4; i++) // 输出上面4行*号
{
for (k = 0; k <= 2 * i - 2; k++) cout << "*"; // 输出*号
cout << endl; // 输出完一行*号后换行
}
for (i = 1; i <= 3; i++) // 输出下面3行*号
{
for (k = 0; k <= 6 - 2 * i; k++) cout << "*"; // 输出*号
cout << endl; // 输出完一行*号后换行
}
return 0;
}
```
以上代码输出的星号图案如下:
```
* * * * *
* * * * *
* * * * *
* * * * *
****** *****
***** ******
**** *******
```
如果你想使用string方法打印星号图案,请提供更多信息或者更具体的问题。
相关推荐
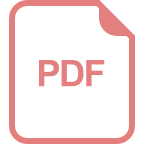
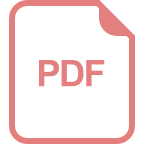
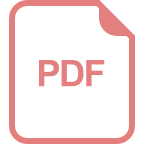









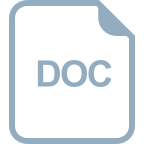