python爬取多网站
时间: 2023-11-24 20:52:35 浏览: 61
以下是在Python中爬取多个网站的方法:
1. 在一个项目中设置多个爬虫,每个爬虫负责爬取一个网站。可以使用Scrapy框架来实现这个功能。
2. 在命令行中输入命令来运行指定的爬虫。例如,使用以下命令来运行名为taobao的爬虫:
```python
scrapy crawl taobao
```
3. 可以使用cmdline模块来在Python文件中运行爬虫。例如,使用以下代码来运行名为taobao、jingdong和其他爬虫的爬虫:
```python
from scrapy import cmdline
cmdline.execute('scrapy crawl taobao'.split())
cmdline.execute('scrapy crawl jingdong'.split())
cmdline.execute('scrapy crawl 其他爬虫'.split())
```
相关问题
Python爬取知乎网站代码
Python爬取知乎网站通常涉及网络请求库如`requests`和解析HTML内容的库如`BeautifulSoup`或`lxml`。以下是一个简单的示例,展示如何用Python爬取知乎文章标题:
```python
import requests
from bs4 import BeautifulSoup
# 定义目标URL
url = "https://www.zhihu.com/topic/19550876/top-answers"
# 发送GET请求获取网页内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 找到包含文章标题的部分(这里假设是h2标签)
article_titles = soup.find_all('h2', class_='QuestionItem-title')
for title in article_titles:
print(title.get_text()) # 输出文章标题
else:
print("请求失败,状态码:", response.status_code)
Python爬取天气网站数据
Python是一种非常适合用于网络爬虫的数据抓取语言,其简洁易读的语法和强大的第三方库如BeautifulSoup、Requests或Scrapy等使其在爬取天气网站数据方面非常流行。以下是使用Python爬取天气网站数据的基本步骤:
1. **安装必要的库**:
- `requests`:发送HTTP请求获取网页内容。
- `beautifulsoup4` 或 `lxml`:解析HTML文档,提取所需信息。
```bash
pip install requests beautifulsoup4
```
2. **发送GET请求**:
使用`requests.get()`函数从目标URL获取网页源码。
```python
import requests
url = "https://example.com/weather" # 替换为你想爬取的天气网站URL
response = requests.get(url)
```
3. **解析HTML**:
使用BeautifulSoup解析响应内容,定位到包含天气信息的部分。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
weather_data = soup.find('div', {'class': 'weather-data'}) # 根据实际页面结构选择合适的标签
```
4. **提取数据**:
找到数据所在的元素并提取出你需要的信息,例如温度、日期等。
```python
temperature = weather_data.find('span', {'class': 'temperature'}).text
date = weather_data.find('span', {'class': 'date'}).text
```
5. **处理和存储数据**:
将提取的数据保存到文件或数据库,可以使用pandas库来方便地操作数据。
```python
import pandas as pd
data = {'Temperature': [temperature], 'Date': [date]}
df = pd.DataFrame(data)
df.to_csv('weather.csv') # 或者使用db连接直接存储到数据库
```
阅读全文
相关推荐
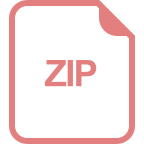
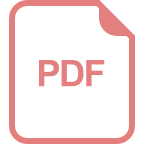
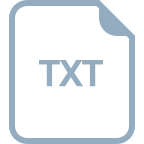













